Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table tblFiles with the schema as follows.
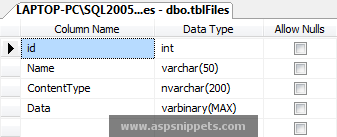
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Data.SqlClient;
using System.IO;
using System.Text;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Hosting;
Controller
public class HomeController : Controller
{
private IHostingEnvironment Environment;
public HomeController(IHostingEnvironment _environment)
{
Environment = _environment;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult Index(IFormFile postedFile)
{
string path = Path.Combine(this.Environment.WebRootPath, "Uploads");
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
string fileName = Path.GetFileName(postedFile.FileName);
using (FileStream stream = new FileStream(Path.Combine(path, fileName), FileMode.Create))
{
postedFile.CopyTo(stream);
}
string contentType = postedFile.ContentType;
byte[] bytes = System.IO.File.ReadAllBytes(Path.Combine(path, fileName));
string constr = @"Server=.\SQL2014;DataBase=dbFiles;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(constr))
{
string query = "insert into tblFiles values (@Name, @ContentType, @Data)";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Name", fileName);
cmd.Parameters.AddWithValue("@ContentType", contentType);
cmd.Parameters.AddWithValue("@Data", bytes);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
return View();
}
[HttpPost]
public IActionResult Read()
{
string path = Path.Combine(this.Environment.WebRootPath, "Files");
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
string name = "";
string constr = @"Server=.\SQL2014;DataBase=dbFiles;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandText = "SELECT Id, Name, Data FROM tblFiles";
cmd.Connection = con;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
if (sdr.Read())
{
name = sdr["Name"].ToString();
System.IO.File.WriteAllBytes(Path.Combine(path, sdr["Name"].ToString()), (byte[])sdr["Data"]);
}
con.Close();
}
}
if (System.IO.File.Exists(Path.Combine(path, name)))
{
string[] texts = System.IO.File.ReadAllLines(Path.Combine(path, name));
StringBuilder sb = new StringBuilder();
foreach (string text in texts)
{
if (text.Contains("to"))
{
sb.Append(text);
sb.Append("<br/>");
}
}
ViewBag.Message = sb.ToString();
}
return View("Index");
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" enctype="multipart/form-data" asp-controller="Home">
<input type="file" name="postedFile" />
<input type="submit" value="Upload" asp-action="Index" />
<input type="submit" value="Read" asp-action="Read" />
<br />
<span style="color:green">@Html.Raw(ViewBag.Message)</span>
</form>
</body>
</html>
Screenshots
Text File

Output
