Hi Ramco,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
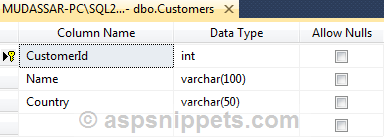
I have already inserted few records in the table.
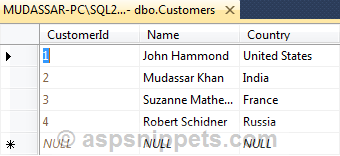
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:Repeater ID="rptCustomers" runat="server">
<HeaderTemplate>
<table>
<tr>
<th>Customer ID:</th>
<th>Name:</th>
<th>Country:</th>
<th></th>
</tr>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td><asp:Label ID="lblId" Text='<%# Eval("CustomerId") %>' runat="server" ></asp:Label></td>
<td><asp:Label ID="lblName" Text='<%# Eval("Name") %>' runat="server"></asp:Label></td>
<td><asp:Label ID="lblCountry" Text='<%# Eval("Country") %>' runat="server"></asp:Label></td>
<td><asp:LinkButton Text="Edit" runat="server" OnClick="Edit"></asp:LinkButton>
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
<tr>
<td><asp:TextBox ID="txtId" runat="server" Width="50px"></asp:TextBox></td>
<td><asp:TextBox ID="txtName" runat="server"></asp:TextBox></td>
<td><asp:TextBox ID="txtCountry" runat="server"></asp:TextBox></td>
<td><asp:LinkButton ID="lnkUpdate" Text="Update" Visible="false" runat="server" OnClick="Update"></asp:LinkButton>
</td>
</tr>
</table>
</FooterTemplate>
</asp:Repeater>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindRepeater();
}
}
private void BindRepeater()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
this.rptCustomers.DataSource = dt;
this.rptCustomers.DataBind();
}
}
}
}
}
protected void Edit(object sender, EventArgs e)
{
RepeaterItem item = (sender as LinkButton).NamingContainer as RepeaterItem;
string id = (item.FindControl("lblId") as Label).Text;
string name = (item.FindControl("lblName") as Label).Text;
string country = (item.FindControl("lblCountry") as Label).Text;
(rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtId") as TextBox).Text = id;
(rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtName") as TextBox).Text = name;
(rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtCountry") as TextBox).Text = country;
(rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("lnkUpdate") as LinkButton).Visible = true;
}
protected void Update(object sender, EventArgs e)
{
LinkButton lnkUpdate = (rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("lnkUpdate") as LinkButton);
int id = int.Parse((rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtId") as TextBox).Text);
string name = (rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtName") as TextBox).Text;
string country = (rptCustomers.Controls[rptCustomers.Controls.Count - 1].Controls[0].FindControl("txtCountry") as TextBox).Text;
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("UPDATE Customers SET Name = @Name, Country = @Country WHERE CustomerId = @Id", con))
{
cmd.CommandType = CommandType.Text;
cmd.Parameters.AddWithValue("@Id", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
this.BindRepeater();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindRepeater()
End If
End Sub
Private Sub BindRepeater()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
Me.rptCustomers.DataSource = dt
Me.rptCustomers.DataBind()
End Using
End Using
End Using
End Using
End Sub
Protected Sub Edit(ByVal sender As Object, ByVal e As EventArgs)
Dim item As RepeaterItem = TryCast((TryCast(sender, LinkButton)).NamingContainer, RepeaterItem)
Dim id As String = (TryCast(item.FindControl("lblId"), Label)).Text
Dim name As String = (TryCast(item.FindControl("lblName"), Label)).Text
Dim country As String = (TryCast(item.FindControl("lblCountry"), Label)).Text
TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtId"), TextBox).Text = id
TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtName"), TextBox).Text = name
TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtCountry"), TextBox).Text = country
TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("lnkUpdate"), LinkButton).Visible = True
End Sub
Protected Sub Update(ByVal sender As Object, ByVal e As EventArgs)
Dim lnkUpdate As LinkButton = (TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("lnkUpdate"), LinkButton))
Dim id As Integer = Integer.Parse((TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtId"), TextBox)).Text)
Dim name As String = (TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtName"), TextBox)).Text
Dim country As String = (TryCast(rptCustomers.Controls(rptCustomers.Controls.Count - 1).Controls(0).FindControl("txtCountry"), TextBox)).Text
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("UPDATE Customers SET Name = @Name, Country = @Country WHERE CustomerId = @Id", con)
cmd.CommandType = CommandType.Text
cmd.Parameters.AddWithValue("@Id", id)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
Me.BindRepeater()
End Sub
Screenshot
