Hi fadi,
Check the below code.
Database
I have made use of the following table Customers with the schema as follows.
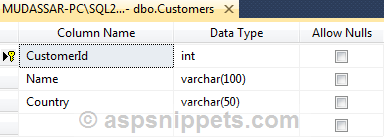
I have already inserted few records in the table.
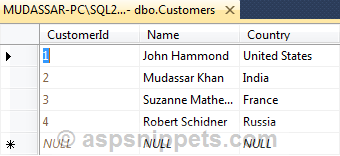
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div id="MyApp">
<div class="site-section bg-light">
<div class="container">
<div class="row">
<div class="col-md-7 mb-5" data-aos="fade">
<form action="#" class="p-5 bg-white">
<div class="row form-group">
<div class="col-md-6 mb-3 mb-md-0">
<input type="text" v-model="Id" placeholder="Enter your Id" class="form-control rounded" />
</div>
</div>
<div class="row form-group">
<div class="col-md-6 mb-3 mb-md-0">
<input type="text" v-model="Name" placeholder="Enter your Name" class="form-control rounded" />
</div>
</div>
<div class="row form-group">
<div class="col-md-6 mb-3 mb-md-0">
<input type="text" v-model="Country" placeholder="Enter your Country" class="form-control rounded" />
</div>
</div>
<div class="row form-group">
<div class="col-md-6 mb-3 mb-md-0">
<input type="button" value="Submit" v-on:click="OnSubmit()" class="btn btn-primary py-2 px-4 text-white" />
</div>
</div>
<br />
</form>
<br />
Id: <span>{{Id}} </span>
<br />
Name: <span>{{Name}} </span>
<br />
Country: <span>{{Country}} </span>
</div>
</div>
</div>
</div>
</div>
<script type="text/javascript" src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css" />
<script type="text/javascript">
var app = new Vue({
el: '#MyApp',
data: {
Id: '',
Name: '',
Country: ''
},
methods: {
OnSubmit: function () {
let currentins = this;
let user = {
Id: this.Id,
Name: this.Name,
Country: this.Country
};
axios({
method: 'post',
url: "Default.aspx/GetData",
data: user
}).then(function (data) {
currentins.Id = (data.d.Id)
currentins.Name = (data.d.Name)
currentins.Country = (data.d.Country)
}).catch(function (err) {
alert(err);
})
}
}
});
</script>
Namespaces
using System.Configuration;
using System.Data.SqlClient;
Code
public Customer GetData(string id)
{
Customer customer = new Customer();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT * FROM Customers WHERE CustomerId = @Id";
using (SqlConnection con = new SqlConnection(conString))
{
SqlCommand cmd = new SqlCommand(query, con);
cmd.Parameters.AddWithValue("@Id", id);
SqlDataReader sdr = cmd.ExecuteReader();
if (sdr.Read())
{
customer.Id = Convert.ToInt32(sdr["CustomerId"]);
customer.Name = Convert.ToString(sdr["Name"]);
customer.Country = Convert.ToString(sdr["Country"]);
}
}
return customer;
}
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
For more details refer below links.
https://www.linkedin.com/pulse/post-data-from-vuejs-aspnet-core-using-axios-adeyinka-oluwaseun
http://aspsolution.net/Code/1/5096/Example-how-to-Post-Data-From-VueJs-In-ASPNET-CORE-Using-Axios/