I have added a TextBox to enter App name and a Button
on Button click it will check and display status in Label of the Application Process Name.
C#
Namespaces
using System.Diagnostics;
Code
public bool IsProcessOpen(string name)
{
foreach (Process clsProcess in Process.GetProcesses())
{
if (clsProcess.ProcessName.ToLower().Contains(name.ToLower()))
{
return true;
}
}
return false;
}
private void button1_Click(object sender, EventArgs e)
{
Process thisProc = Process.GetCurrentProcess();
if (IsProcessOpen(this.textBox1.Text.Trim()) == false)
{
string message = string.Format("{0} is not running.", this.textBox1.Text.Trim());
this.lblStatus.Text = message;
}
else
{
string message = string.Format("{0} is running.", this.textBox1.Text.Trim());
this.lblStatus.Text = message;
}
}
VB
Public Function IsProcessOpen(name As String) As Boolean
For Each clsProcess As Process In Process.GetProcesses()
If clsProcess.ProcessName.ToLower().Contains(name.ToLower()) Then
Return True
End If
Next
Return False
End Function
Private Sub button1_Click(sender As System.Object, e As System.EventArgs) Handles button1.Click
Dim thisProc As Process = Process.GetCurrentProcess()
If IsProcessOpen(Me.textBox1.Text.Trim()) = False Then
Dim message As String = String.Format("{0} is not running.", Me.textBox1.Text.Trim())
Me.lblStatus.Text = message
Else
Dim message As String = String.Format("{0} is running.", Me.textBox1.Text.Trim())
Me.lblStatus.Text = message
End If
End Sub
Screenshots
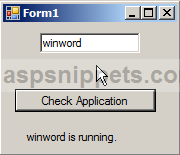
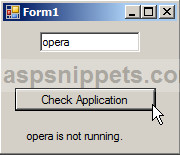