Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
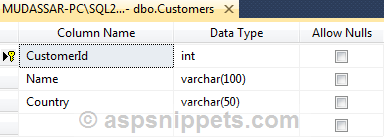
I have already inserted few records in the table.
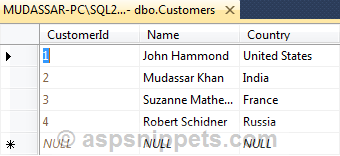
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using System.Web.Services;
Code
[WebMethod]
public static List<object> GetCustomers()
{
List<object> customers = new List<object>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT * FROM Customers";
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
cmd.Connection = con;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
customers.Add(new
{
Id = Convert.ToInt32(sdr["CustomerId"]),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
con.Close();
}
return customers;
}
[WebMethod]
public static void AddUpdateCustomer(int id, string name, string country)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "";
SqlCommand cmd = new SqlCommand();
if (id > 0)
{
query = "UPDATE Customers SET Name = @Name,Country = @Country WHERE CustomerId = @Id";
cmd.Parameters.AddWithValue("@Id", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
}
else
{
query = "INSERT INTO Customers VALUES (@Name, @Country)";
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
}
using (SqlConnection con = new SqlConnection(conString))
{
cmd.CommandText = query;
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
[WebMethod]
public static void DeleteCustomer(int id)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "DELETE FROM Customers WHERE CustomerId = @Id";
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Id", id);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
HTML
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.5.5/angular.js"></script>
<script type="text/javascript">
var app = angular.module('MyApp', []);
app.controller('MyController', function ($scope, $http, $window) {
$scope.ButtonText = "Add";
GetCustomers($scope);
$scope.Add = function () {
var obj = {};
obj.id = $scope.Id == undefined ? 0 : $scope.Id;
obj.name = $scope.Name;
obj.country = $scope.Country;
$.ajax({
url: 'Default.aspx/AddUpdateCustomer',
type: 'POST',
contentType: 'application/json',
data: JSON.stringify(obj),
success: function (response) {
GetCustomers($scope);
$scope.Id = "";
$scope.Name = "";
$scope.Country = "";
$scope.ButtonText = "Add";
},
error: function (err) {
alert(response.responseText);
}
});
}
$scope.Delete = function (id) {
var obj = {};
obj.id = id;
$.ajax({
url: 'Default.aspx/DeleteCustomer',
type: "POST",
contentType: 'application/json',
data: JSON.stringify(obj),
success: function (response) {
GetCustomers($scope);
},
error: function (err) {
alert(response.responseText);
}
});
}
$scope.Edit = function (id) {
var customers = $scope.Customers;
customers.map(function (customer) {
if (customer.Id == id) {
$scope.Id = customer.Id;
$scope.Name = customer.Name;
$scope.Country = customer.Country;
$scope.ButtonText = "Update";
}
});
}
});
function GetCustomers($scope) {
$.ajax({
url: 'Default.aspx/GetCustomers',
type: "POST",
contentType: 'application/json',
success: function (response) {
$scope.Customers = response.d;
$scope.$apply();
},
error: function (err) {
alert(response.responseText);
}
});
}
</script>
<div ng-app="MyApp" ng-controller="MyController">
<div class="container">
<div class="row">
<table class="table">
<tr>
<td>Name</td>
<td>
<input type="hidden" ng-model="Id" />
<input type="text" id="txtName" class="form-control" name="name" ng-model="Name" />
</td>
</tr>
<tr>
<td>Country</td>
<td><input type="text" id="txtCountry" class="form-control" name="country" ng-model="Country" /></td>
</tr>
<tr>
<td colspan="2" align="center">
<button type="button" id="btnInsert" class="btn btn-primary" ng-click="Add()">{{ButtonText}}</button>
</td>
</tr>
</table>
</div>
</div>
<div class="container">
<div class="row">
<table class="table table-striped table-bordered table-condensed">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
<th>Edit | Delete</th>
</tr>
</thead>
<tbody ng-repeat="customer in Customers">
<tr>
<td>{{customer.Id}}</td>
<td>{{customer.Name}}</td>
<td>{{customer.Country}}</td>
<td>
<button type="button" id="btnEdit" class="btn btn-primary" ng-click="Edit(customer.Id)"><i class="fa fa-edit"></i></button>
<button type="button" id="btnDelete" class="btn btn-primary" ng-click="Delete(customer.Id)"><i class="fa fa-trash-o"></i></button>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
Screenshot
