Hi akhter,
Check the below example.
Database
I have used two tables Countries and State are created with the following schema.
Countries Table
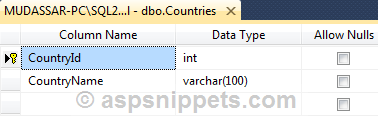
States Table
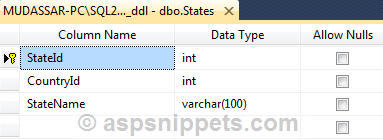
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CascadingModel
{
public int CountryId { get; set; }
public int StateId { get; set; }
public SelectList Countries { get; set; }
public SelectList States { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Data.Entity.SqlServer;
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
CascadingModel model = new CascadingModel();
model.Countries = new SelectList(PopulateCountries(), "Value", "Text");
model.States = new SelectList(PopulateStates(), "Value", "Text");
return View(model);
}
[HttpPost]
public JsonResult GetStates(string param)
{
return Json(PopulateStates(Convert.ToInt32(param)));
}
[HttpPost]
public ActionResult Index(int countryId, int stateId)
{
CascadingModel model = new CascadingModel();
model.Countries = new SelectList(PopulateCountries(), "Value", "Text");
model.States = new SelectList(PopulateStates(countryId), "Value", "Text");
return View(model);
}
private static List<SelectListItem> PopulateCountries()
{
CascadingEntities entities = new CascadingEntities();
List<SelectListItem> countries = (from d in entities.Countries
select new SelectListItem
{
Text = d.CountryName,
Value = SqlFunctions.StringConvert((double)d.CountryId)
}).ToList();
countries.Insert(0, new SelectListItem { Text = "--Select Country--", Value = "" });
return countries;
}
private static List<SelectListItem> PopulateStates(int countryId = 0)
{
CascadingEntities entities = new CascadingEntities();
List<SelectListItem> states = new List<SelectListItem>();
if (countryId > 0)
{
states = (from d in entities.States.Where(x => x.CountryId == countryId)
select new SelectListItem
{
Text = d.StateName,
Value = SqlFunctions.StringConvert((double)d.StateId),
Selected = d.StateName == "Maharashtra" ? true : false // Set the selected.
}).ToList();
}
else
{
states = (from d in entities.States
select new SelectListItem
{
Text = d.StateName,
Value = SqlFunctions.StringConvert((double)d.StateId)
}).ToList();
}
states.Insert(0, new SelectListItem { Text = "--Select State--", Value = "" });
return states;
}
}
View
@model Cascading_DropDownList_MVC.Models.CascadingModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
@Html.DropDownListFor(m => m.CountryId, (IEnumerable<SelectListItem>)Model.Countries, new { @class = "form-control" })
<br />
<br />
@Html.DropDownListFor(m => m.StateId, (IEnumerable<SelectListItem>)Model.States, new { @class = "form-control" })
<br />
<br />
<input type="submit" value="Submit" />
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script type="text/javascript">
$(function () {
$('#CountryId').change(function () {
var value = 0;
if ($(this).val() != "") {
value = $(this).val();
}
$.ajax({
type: "POST",
url: "/Home/GetStates",
data: '{param: "' + value + '"}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
var list = response;
$("#StateId").empty();
PopulateDropDown("#StateId", list);
},
error: function (response) {
alert(response.responseText);
}
});
});
});
function PopulateDropDown(dropDownId, list) {
if (list != null && list.length > 0) {
$(dropDownId).removeAttr("disabled");
$.each(list, function () {
if (this['Selected']) {
$(dropDownId).append($("<option selected='selected'></option>").val(this['Value']).html(this['Text']));
} else {
$(dropDownId).append($("<option></option>").val(this['Value']).html(this['Text']));
}
});
}
}
$(function () {
if ($('#CountryId').val() == "") {
$("#StateId").prop("disabled", true);
}
if ($("#CountryId").val() != "" && $("#StateId").val() != "") {
var message = "Country: " + $("#CountryId option:selected").text();
message += "\nState: " + $("#StateId option:selected").text();
alert(message);
}
});
</script>
</body>
</html>
Screenshot
