Hi akhter,
I have created a sample please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
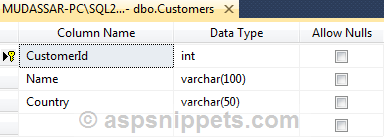
I have already inserted few records in the table.
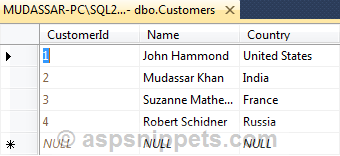
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div style="height: 800px; width: 950px; overflow: auto;">
<asp:ScriptManager EnablePartialRendering="true" ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="False" OnRowEditing="gvso_RowEditing"
OnRowUpdating="gvso_RowUpdating" OnRowCancelingEdit="gvso_RowCancelingEdit">
<Columns>
<asp:TemplateField HeaderText="Customer Id">
<ItemTemplate>
<%# Eval("CustomerId") %>
</ItemTemplate>
<EditItemTemplate>
<asp:Label ID="lblId" runat="server" Text='<%#Eval("CustomerId") %>'></asp:Label>
</EditItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<EditItemTemplate>
<asp:TextBox ID="txtName" runat="server" Text='<%# Eval("Name") %>'></asp:TextBox>
</EditItemTemplate>
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Eval("Name") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country">
<EditItemTemplate>
<asp:TextBox ID="txtCountry" runat="server" Text='<%# Eval("Country") %>'></asp:TextBox>
</EditItemTemplate>
<ItemTemplate>
<asp:Label ID="lblCountry" runat="server" Text='<%# Eval("Country") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="true" HeaderText="Edit" />
</Columns>
</asp:GridView>
</ContentTemplate>
</asp:UpdatePanel>
</div>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data.SqlClient
Imports System.Data
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
BindGrid();
}
}
private void BindGrid()
{
using (SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["conString"].ConnectionString))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerID,Name,Country FROM Customers", con))
{
cmd.CommandType = CommandType.Text;
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
DataTable dt = new DataTable();
sda.Fill(dt);
this.gvCustomers.DataSource = dt;
this.gvCustomers.DataBind();
}
}
}
}
protected void gvso_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
string id = ((Label)(gvCustomers.Rows[e.RowIndex].FindControl("lblId"))).Text;
string name = ((TextBox)(gvCustomers.Rows[e.RowIndex].FindControl("txtName"))).Text;
string country = (((TextBox)(gvCustomers.Rows[e.RowIndex].FindControl("txtCountry"))).Text);
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["conString"].ConnectionString);
con.Open();
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "UPDATE Customers SET Name=@Name,Country=@Country WHERE CustomerId=@CustomerId";
cmd.Parameters.AddWithValue("@CustomerId", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
cmd.Connection = con;
cmd.ExecuteNonQuery();
gvCustomers.EditIndex = -1;
this.BindGrid();
}
protected void gvso_RowEditing(object sender, GridViewEditEventArgs e)
{
this.gvCustomers.EditIndex = e.NewEditIndex;
this.BindGrid();
}
protected void gvso_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
this.gvCustomers.EditIndex = -1;
this.BindGrid();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
BindGrid()
End If
End Sub
Private Sub BindGrid()
Using con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("conString").ConnectionString)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerID,Name,Country FROM Customers", con)
cmd.CommandType = CommandType.Text
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Dim dt As DataTable = New DataTable()
sda.Fill(dt)
Me.gvCustomers.DataSource = dt
Me.gvCustomers.DataBind()
End Using
End Using
End Using
End Sub
Protected Sub gvso_RowUpdating(ByVal sender As Object, ByVal e As GridViewUpdateEventArgs)
Dim id As String = (CType((gvCustomers.Rows(e.RowIndex).FindControl("lblId")), Label)).Text
Dim name As String = (CType((gvCustomers.Rows(e.RowIndex).FindControl("txtName")), TextBox)).Text
Dim country As String = ((CType((gvCustomers.Rows(e.RowIndex).FindControl("txtCountry")), TextBox)).Text)
Dim con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("conString").ConnectionString)
con.Open()
Dim cmd As SqlCommand = New SqlCommand()
cmd.CommandText = "UPDATE Customers SET Name=@Name,Country=@Country WHERE CustomerId=@CustomerId"
cmd.Parameters.AddWithValue("@CustomerId", id)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
cmd.Connection = con
cmd.ExecuteNonQuery()
gvCustomers.EditIndex = -1
Me.BindGrid()
End Sub
Protected Sub gvso_RowEditing(ByVal sender As Object, ByVal e As GridViewEditEventArgs)
Me.gvCustomers.EditIndex = e.NewEditIndex
Me.BindGrid()
End Sub
Protected Sub gvso_RowCancelingEdit(ByVal sender As Object, ByVal e As GridViewCancelEditEventArgs)
Me.gvCustomers.EditIndex = -1
Me.BindGrid()
End Sub
Screenshot
