Hi SajidHussa,
Refer below code.
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
[HttpPost]
public ActionResult Index(string ddlCustomers)
{
List<SelectListItem> customerList = GetCustomers();
if (!string.IsNullOrEmpty(ddlCustomers))
{
SelectListItem selectedItem = customerList.Find(p => p.Value == ddlCustomers);
ViewBag.Message = "Name: " + selectedItem.Text;
ViewBag.Message += "\\nID: " + selectedItem.Value;
}
return View(customerList);
}
[HttpPost]
public JsonResult AjaxMethod()
{
return Json(GetCustomers());
}
private List<SelectListItem> GetCustomers()
{
List<SelectListItem> customers = new List<SelectListItem>();
customers.Add(new SelectListItem { Value = "1", Text = "John Hammond" });
customers.Add(new SelectListItem { Value = "2", Text = "Mudassar Khan" });
customers.Add(new SelectListItem { Value = "3", Text = "Suzanne Mathews" });
customers.Add(new SelectListItem { Value = "4", Text = "Robert Schidner" });
return customers;
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
<select id="ddlCustomers" name="ddlCustomers"></select>
<br /><br />
<input type="submit" value="Submit" />
}
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/css/select2.min.css" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/js/select2.min.js"></script>
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
url: "/Home/AjaxMethod",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
$("#ddlCustomers").empty().append('<option selected="selected" value="0">Please select</option>');
$.each(response, function () {
$("#ddlCustomers").append($("<option></option>").val(this['Value']).html(this['Text']));
});
$("[id*=ddlCustomers]").select2();
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
});
</script>
</body>
</html>
Screenshot
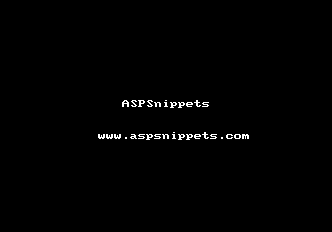