Hi SUJAYS,
Refer below example.
For reading connection string refer below article.
Database
I have made use of table Customers with the schema as follows. CustomerId is an Auto-Increment (Identity) column.
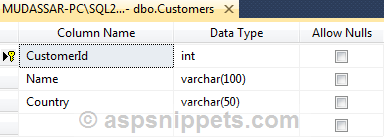
I have already inserted few records in the table.
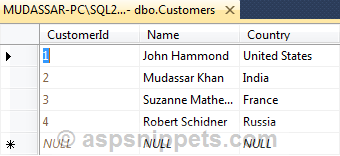
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Data;
using System.Data.SqlClient;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
Model
public class Customer
{
public int Id { set; get; }
public string Name { set; get; }
public string Country { set; get; }
public IEnumerable<Customer> Customers { get; set; }
}
Controller
public class HomeController : Controller
{
private IConfiguration Configuration;
public HomeController(IConfiguration _configuration)
{
Configuration = _configuration;
}
private string GetConnectionString()
{
return this.Configuration.GetConnectionString("MyConn");
}
public List<Customer> GetCustomers(int? id)
{
List<Customer> customers = new List<Customer>();
using (SqlConnection con = new SqlConnection(GetConnectionString()))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.Connection = con;
cmd.CommandType = CommandType.Text;
cmd.Parameters.Clear();
if (id != null)
{
cmd.CommandText = "SELECT * FROM Customers WHERE CustomerId = @Id";
cmd.Parameters.AddWithValue("@Id", id);
}
else
{
cmd.CommandText = "SELECT * FROM Customers";
}
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
customers.Add(new Customer()
{
Id = Convert.ToInt32(rdr["CustomerId"]),
Name = rdr["Name"].ToString(),
Country = rdr["Country"].ToString()
});
}
con.Close();
}
}
return customers;
}
public void AddUpdateDeleteCustomer(Customer customer, string action)
{
using (SqlConnection con = new SqlConnection(GetConnectionString()))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
cmd.Parameters.Clear();
if (action == "Insert")
{
cmd.CommandText = "INSERT INTO Customers VALUES (@Name,@Country)";
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
}
else if (action == "Update")
{
cmd.CommandText = "UPDATE Customers SET NAME = @Name,Country = @Country WHERE CustomerId = @Id";
cmd.Parameters.AddWithValue("@Id", customer.Id);
cmd.Parameters.AddWithValue("@Name", customer.Name);
cmd.Parameters.AddWithValue("@Country", customer.Country);
}
else if (action == "Delete")
{
cmd.CommandText = "DELETE FROM Customers WHERE CustomerId = @Id";
cmd.Parameters.AddWithValue("@Id", customer.Id);
}
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
public IActionResult Index()
{
ViewBag.Name = "Add";
Customer customer = new Customer();
customer.Customers = GetCustomers(null);
return View(customer);
}
public IActionResult Create(Customer customer)
{
AddUpdateDeleteCustomer(customer, "Insert");
return RedirectToAction("Index");
}
public IActionResult Edit(int id)
{
ViewBag.Name = "Update";
Customer customer = new Customer();
customer.Id = id;
customer.Name = GetCustomers(id).FirstOrDefault().Name;
customer.Country = GetCustomers(id).FirstOrDefault().Country;
customer.Customers = GetCustomers(null);
return View("Index", customer);
}
public IActionResult Update(Customer customer)
{
ViewBag.Name = "Add";
AddUpdateDeleteCustomer(customer, "Update");
return RedirectToAction("Index");
}
public IActionResult Delete(int id)
{
ViewBag.Name = "Add";
AddUpdateDeleteCustomer(new Customer { Id = id }, "Delete");
return RedirectToAction("Index");
}
}
View
Index
@model ASP.Net_Core_MVC_CRUD.Models.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<link href="~/lib/bootstrap/dist/css/bootstrap.css" rel="stylesheet" />
</head>
<body>
<div class="container">
<div class="row">
<div class="col-lg-12">
<h4>Customers</h4>
<table class="table table-responsive">
<thead>
<tr>
<th>@Html.DisplayNameFor(model => model.Name)</th>
<th>@Html.DisplayNameFor(model => model.Country)</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (Customer customer in Model.Customers)
{
<tr>
<td>@Html.DisplayFor(x => customer.Name)</td>
<td>@Html.DisplayFor(x => customer.Country)</td>
<td>@Html.ActionLink("Edit", "Edit", new { id = customer.Id }, new { @class = "btn btn-primary btn-sm" })</td>
<td>@Html.ActionLink("Delete", "Delete", new { id = customer.Id }, new { onclick = "return confirm('Are you sure you wish to delete this Customer?');", @class = "btn btn-danger btn-sm" })</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
@Html.Partial("_InsertUpdatePartial", Model)
</body>
</html>
PartialView
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model ASP.Net_Core_MVC_CRUD.Models.Customer
<div class="container">
<h4>Add/Update Customer</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Create" asp-controller="Home">
<div class="form-group">
<label asp-for="Id" class="control-label"></label>
<input asp-for="Id" class="form-control" readonly="readonly" />
</div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
</div>
<div class="form-group">
<label asp-for="Country" class="control-label"></label>
<input asp-for="Country" class="form-control" />
</div>
<div class="form-group">
@if (ViewBag.Name == "Add")
{
<input type="submit" value="Add" class="btn btn-success btn-sm" />
}
else
{
<input type="submit" value="Update" asp-action="Update" class="btn btn-success btn-sm" />
<input type="submit" value="Cancel" asp-action="Index" class="btn btn-defaul btn-sm" />
}
</div>
</form>
</div>
</div>
</div>
Screenshot
