Hi paulrajmca,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table tblFiles with the schema as follows.
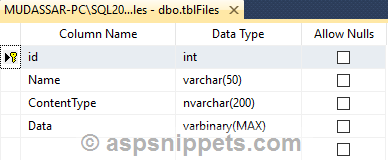
Download SQL file
HTML
<asp:FileUpload ID="fuUpload" runat="server" />
<asp:Button Text="Save" runat="server" ID="btnSave" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
var reader = new FileReader();
var fileName, type;
$('[id*=fuUpload]').change(function () {
var file = $(this)[0].files;
if (typeof (FileReader) != "undefined") {
fileName = file[0].name;
type = file[0].type;
reader.readAsDataURL(file[0]);
} else {
alert("This browser does not support HTML5 FileReader.");
}
});
$("[id*=btnSave]").click(function () {
var byteData = reader.result;
byteData = byteData.split(';')[1].replace("base64,", "");
var obj = {};
obj.Data = byteData;
obj.Name = fileName;
obj.Type = type;
$.ajax({
type: "POST",
url: "FileService.asmx/SaveFile",
data: '{fileData : ' + JSON.stringify(obj) + ' }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
if (r.d == 1) {
alert("File Uploaded successfully.");
}
},
error: function (r) {
alert(r.responseText);
}
});
});
});
</script>
WebService
C#
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Linq;
using System.Web;
using System.Web.Services;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class FileService : System.Web.Services.WebService
{
[WebMethod]
public int SaveFile(FileData fileData)
{
int i = 0;
if (!string.IsNullOrEmpty(fileData.Name))
{
byte[] fileBytes = Convert.FromBase64String(fileData.Data);
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection conn = new SqlConnection(constr))
{
string sql = "INSERT INTO tblFiles VALUES(@Name, @ContentType, @Data)";
using (SqlCommand cmd = new SqlCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@Name", fileData.Name);
cmd.Parameters.AddWithValue("@ContentType", fileData.Type);
cmd.Parameters.AddWithValue("@Data", fileBytes);
conn.Open();
i = cmd.ExecuteNonQuery();
conn.Close();
}
}
}
return i;
}
public class FileData
{
public string Name { get; set; }
public string Data { get; set; }
public string Type { get; set; }
}
}
VB.Net
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web
Imports System.Web.Services
Imports System.Web.Services.Protocols
' To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
<System.Web.Script.Services.ScriptService()>
<WebService(Namespace:="http://tempuri.org/")>
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)>
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()>
Public Class FileService
Inherits System.Web.Services.WebService
<WebMethod>
Public Function SaveFile(ByVal fileData As FileData) As Integer
Dim i As Integer = 0
If Not String.IsNullOrEmpty(fileData.Name) Then
Dim fileBytes As Byte() = Convert.FromBase64String(fileData.Data)
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using conn As SqlConnection = New SqlConnection(constr)
Dim sql As String = "INSERT INTO tblFiles VALUES(@Name, @ContentType, @Data)"
Using cmd As SqlCommand = New SqlCommand(sql, conn)
cmd.Parameters.AddWithValue("@Name", fileData.Name)
cmd.Parameters.AddWithValue("@ContentType", fileData.Type)
cmd.Parameters.AddWithValue("@Data", fileBytes)
conn.Open()
i = cmd.ExecuteNonQuery()
conn.Close()
End Using
End Using
End If
Return i
End Function
Public Class FileData
Public Property Name As String
Public Property Data As String
Public Property Type As String
End Class
End Class