Hi rani,
Using the below article i have created the example.
Fo passing DataTable to View i have used DataTable as model for the View.
Check this example. Now please take its reference and correct your code.
Namespaces
using Microsoft.Extensions.Configuration;
using System.Data;
using System.Data.OleDb;
using System.IO;
Controller
public class HomeController : Controller
{
private IHostingEnvironment Environment;
private IConfiguration Configuration;
public HomeController(IHostingEnvironment _environment, IConfiguration _configuration)
{
Environment = _environment;
Configuration = _configuration;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult Index(IFormFile postedFile)
{
if (postedFile != null)
{
string path = Path.Combine(this.Environment.WebRootPath, "Uploads");
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
string fileName = Path.GetFileName(postedFile.FileName);
string filePath = Path.Combine(path, fileName);
using (FileStream stream = new FileStream(filePath, FileMode.Create))
{
postedFile.CopyTo(stream);
}
string conString = this.Configuration.GetConnectionString("ExcelConString");
DataTable dt = new DataTable();
conString = string.Format(conString, filePath);
using (OleDbConnection connExcel = new OleDbConnection(conString))
{
using (OleDbCommand cmdExcel = new OleDbCommand())
{
using (OleDbDataAdapter odaExcel = new OleDbDataAdapter())
{
cmdExcel.Connection = connExcel;
connExcel.Open();
DataTable dtExcelSchema;
dtExcelSchema = connExcel.GetOleDbSchemaTable(OleDbSchemaGuid.Tables, null);
string sheetName = dtExcelSchema.Rows[0]["TABLE_NAME"].ToString();
connExcel.Close();
connExcel.Open();
cmdExcel.CommandText = "SELECT * From [" + sheetName + "]";
odaExcel.SelectCommand = cmdExcel;
odaExcel.Fill(dt);
connExcel.Close();
}
}
}
return View(dt);
}
return View();
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using System.Data;
@model DataTable
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-controller="Home" asp-action="Index" method="post" enctype="multipart/form-data">
<input type="file" name="postedFile" />
<input type="submit" value="Import" />
</form>
@if (Model != null)
{
<h4>Customers</h4>
<hr />
<table cellpadding="0" cellspacing="0">
<tr>
@foreach (DataColumn column in Model.Columns)
{
<th>
@column.ColumnName
</th>
}
</tr>
@foreach (DataRow row in Model.Rows)
{
<tr>
@foreach (var item in row.ItemArray)
{
<td>@item</td>
}
</tr>
}
</table>
}
</body>
</html>
Screenshot
The excel file
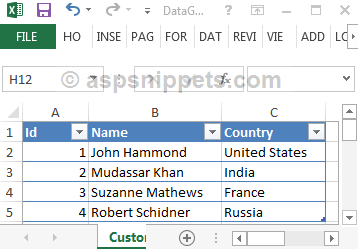
The View
