Hi akhter,
You need to modify your BindGrid method to pass the textbox value as parameter to id.
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
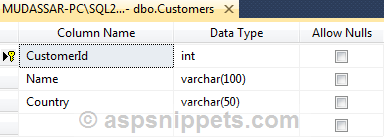
I have already inserted few records in the table.
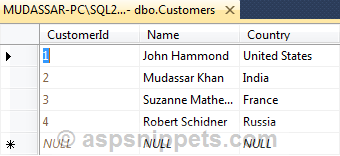
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
Search Customer :
<asp:TextBox ID="txtId" AutoPostBack="true" runat="server" OnTextChanged="Id_TextChanged"></asp:TextBox>
<br />
<%=DateTime.Now %>
<hr />
<div style="height: 800px; width: 670px; overflow: auto;">
<asp:ScriptManager EnablePartialRendering="true" ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<%=DateTime.Now %>
<br />
<asp:GridView ID="gv1" runat="server" AutoGenerateColumns="False" OnRowEditing="gv1_RowEditing"
DataKeyNames="CustomerId" ShowFooter="false" OnRowUpdating="gv1_RowUpdating"
OnRowCancelingEdit="gv1_RowCancelingEdit">
<Columns>
<asp:TemplateField HeaderText="CustomerId">
<ItemTemplate>
<%# Eval("CustomerId")%>
</ItemTemplate>
<EditItemTemplate>
<asp:Label ID="lblId" runat="server" Text='<%#Eval("CustomerId") %>'></asp:Label>
</EditItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<%#Eval("Name")%>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<%#Eval("Country")%>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox ID="txtCountry" runat="server" Text='<%#Eval("Country") %>'></asp:TextBox>
</EditItemTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="true" ControlStyle-ForeColor="Blue ">
<ControlStyle ForeColor="Blue"></ControlStyle>
</asp:CommandField>
</Columns>
</asp:GridView>
</ContentTemplate>
</asp:UpdatePanel>
</div>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Code
C#
SqlConnection con = new SqlConnection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGrid(txtId.Text.Trim());
}
}
public void BindGrid(string id)
{
con = new SqlConnection("Server=.;DataBase=Test;UID=sa;PWD=pass@123;");
con.Open();
SqlCommand ocmd = new SqlCommand("SELECT TOP 4 * FROM Customers WHERE CustomerId = @Id OR @Id IS NULL", con);
ocmd.CommandType = CommandType.Text;
if (!string.IsNullOrEmpty(id))
{
ocmd.Parameters.AddWithValue("@Id", id);
}
else
{
ocmd.Parameters.AddWithValue("@Id", (object)DBNull.Value);
}
SqlDataAdapter oda = new SqlDataAdapter(ocmd);
SqlCommandBuilder builder = new SqlCommandBuilder(oda);
DataSet ds = new DataSet();
oda.Fill(ds);
gv1.DataSource = ds;
gv1.DataBind();
con.Close();
}
protected void gv1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
gv1.EditIndex = -1;
BindGrid(txtId.Text.Trim());
}
protected void gv1_RowEditing(object sender, GridViewEditEventArgs e)
{
gv1.EditIndex = e.NewEditIndex;
BindGrid(txtId.Text.Trim());
}
protected void gv1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
int id = int.Parse(((Label)gv1.Rows[e.RowIndex].FindControl("lblId")).Text);
string country = ((TextBox)gv1.Rows[e.RowIndex].FindControl("txtCountry")).Text;
con = new SqlConnection(".;DataBase=Test;UID=sa;PWD=pass@123;");
con.Open();
SqlCommand ocmd = new SqlCommand();
ocmd.CommandText = "UPDATE Customers SET Country=@Country WHERE CustomerId=@Id";
ocmd.Parameters.AddWithValue("@Country", country);
ocmd.Parameters.AddWithValue("@Id", id);
ocmd.Connection = con;
ocmd.ExecuteNonQuery();
gv1.EditIndex = -1;
BindGrid(txtId.Text.Trim());
}
protected void Id_TextChanged(object sender, EventArgs e)
{
BindGrid(txtId.Text.Trim());
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
BindGrid(txtId.Text.Trim())
End If
End Sub
Public Sub BindGrid(ByVal id As String)
con = New SqlConnection("Server=.;DataBase=Test;UID=sa;PWD=pass@123;")
con.Open()
Dim ocmd As SqlCommand = New SqlCommand("SELECT TOP 4 * FROM Customers WHERE CustomerId = @Id OR @Id IS NULL", con)
ocmd.CommandType = CommandType.Text
If Not String.IsNullOrEmpty(id) Then
ocmd.Parameters.AddWithValue("@Id", id)
Else
ocmd.Parameters.AddWithValue("@Id", CObj(DBNull.Value))
End If
Dim oda As SqlDataAdapter = New SqlDataAdapter(ocmd)
Dim builder As SqlCommandBuilder = New SqlCommandBuilder(oda)
Dim ds As DataSet = New DataSet()
oda.Fill(ds)
gv1.DataSource = ds
gv1.DataBind()
con.Close()
End Sub
Protected Sub gv1_RowCancelingEdit(ByVal sender As Object, ByVal e As GridViewCancelEditEventArgs)
gv1.EditIndex = -1
BindGrid(txtId.Text.Trim())
End Sub
Protected Sub gv1_RowEditing(ByVal sender As Object, ByVal e As GridViewEditEventArgs)
gv1.EditIndex = e.NewEditIndex
BindGrid(txtId.Text.Trim())
End Sub
Protected Sub gv1_RowUpdating(ByVal sender As Object, ByVal e As GridViewUpdateEventArgs)
Dim id As Integer = Integer.Parse((CType(gv1.Rows(e.RowIndex).FindControl("lblId"), Label)).Text)
Dim country As String = (CType(gv1.Rows(e.RowIndex).FindControl("txtCountry"), TextBox)).Text
con = New SqlConnection("Server=.;DataBase=Test;UID=sa;PWD=pass@123;")
con.Open()
Dim ocmd As SqlCommand = New SqlCommand()
ocmd.CommandText = "UPDATE Customers SET Country=@Country WHERE CustomerId=@Id"
ocmd.Parameters.AddWithValue("@Country", country)
ocmd.Parameters.AddWithValue("@Id", id)
ocmd.Connection = con
ocmd.ExecuteNonQuery()
gv1.EditIndex = -1
BindGrid(txtId.Text.Trim())
End Sub
Protected Sub Id_TextChanged(ByVal sender As Object, ByVal e As EventArgs)
BindGrid(txtId.Text.Trim())
End Sub
Screenshot
