Hi themonksden,
To resolve this issue, you need to hide the GridView by setting the Style display to none and show it in initComplete callback function.
initComplete let you know when the data is fully loaded.
For more details on initComplete refer following link.
https://datatables.net/reference/option/initComplete
Using the following article i have modified the sample.
HTML
The HTML Markup consists of GridView control with 4 BoundField columns.
The GridView has been set with Style display: none.
<div style="width: 500px">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" Style="display: none">
<Columns>
<asp:BoundField DataField="CustomerID" HeaderText="Customer Id" />
<asp:BoundField DataField="ContactName" HeaderText="Name" />
<asp:BoundField DataField="City" HeaderText="City" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
</div>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.20/js/jquery.dataTables.min.js"></script>
<link href="https://cdn.datatables.net/1.10.20/css/jquery.dataTables.css" rel="stylesheet" type="text/css" />
<script type="text/javascript">
$(function () {
$("[id*=GridView1]").DataTable({
bLengthChange: true,
lengthMenu: [[5, 10, -1], [5, 10, "All"]],
bFilter: true,
bSort: true,
bPaginate: true,
initComplete: function () {
// Show GridView.
$("[id*=GridView1]").show();
}
});
});
</script>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid();
}
}
private void BindGrid()
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlDataAdapter sda = new SqlDataAdapter("SELECT CustomerID, ContactName, City, Country FROM Customers", con))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
//Required for jQuery DataTables to work.
GridView1.UseAccessibleHeader = true;
GridView1.HeaderRow.TableSection = TableRowSection.TableHeader;
}
VB.Net
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid()
End If
End Sub
Private Sub BindGrid()
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As New SqlConnection(constr)
Using sda As New SqlDataAdapter("SELECT CustomerID, ContactName, City, Country FROM Customers", con)
Using dt As New DataTable()
sda.Fill(dt)
GridView1.DataSource = dt
GridView1.DataBind()
End Using
End Using
End Using
'Required for jQuery DataTables to work.
GridView1.UseAccessibleHeader = True
GridView1.HeaderRow.TableSection = TableRowSection.TableHeader
End Sub
Screenshot
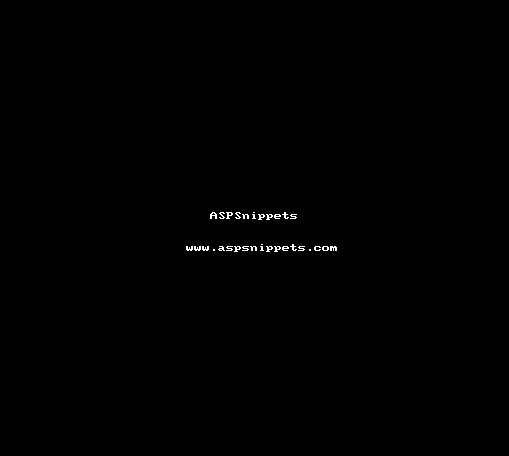