Hi Ramco,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
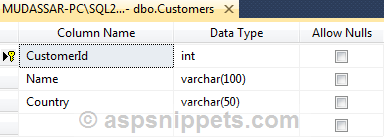
I have already inserted few records in the table.
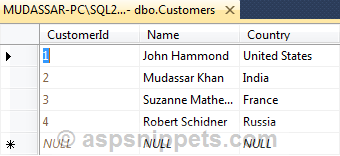
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="Customer Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
Namespaces
C#
using System.Data;
using System.Reflection;
VB.Net
Imports System.Data
Imports System.Reflection
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
AjaxSamplesEntities entity = new AjaxSamplesEntities();
var customers = (from customer in entity.Customers
select customer);
DataTable dt = this.LINQResultToDataTable(customers);
gvCustomers.DataSource = dt;
gvCustomers.DataBind();
}
}
public DataTable LINQResultToDataTable<T>(IEnumerable<T> items)
{
DataTable dataTable = new DataTable(typeof(T).Name);
PropertyInfo[] Props = typeof(T).GetProperties(BindingFlags.Public | BindingFlags.Instance);
foreach (PropertyInfo prop in Props)
{
Type colType = prop.PropertyType;
dataTable.Columns.Add(new DataColumn(prop.Name, colType));
}
foreach (T item in items)
{
var values = new object[Props.Length];
for (int i = 0; i < Props.Length; i++)
{
values[i] = Props[i].GetValue(item, null);
}
dataTable.Rows.Add(values);
}
return dataTable;
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim entity As AjaxSamplesEntities = New AjaxSamplesEntities()
Dim customers = (From customer In entity.Customers Select customer)
Dim dt As DataTable = Me.LINQResultToDataTable(customers)
gvCustomers.DataSource = dt
gvCustomers.DataBind()
End If
End Sub
Public Function LINQResultToDataTable(Of T)(ByVal items As IEnumerable(Of T)) As DataTable
Dim dataTable As DataTable = New DataTable(GetType(T).Name)
Dim Props As PropertyInfo() = GetType(T).GetProperties(BindingFlags.[Public] Or BindingFlags.Instance)
For Each prop As PropertyInfo In Props
Dim colType As Type = prop.PropertyType
dataTable.Columns.Add(New DataColumn(prop.Name, colType))
Next
For Each item As T In items
Dim values = New Object(Props.Length - 1) {}
For i As Integer = 0 To Props.Length - 1
values(i) = Props(i).GetValue(item, Nothing)
Next
dataTable.Rows.Add(values)
Next
Return dataTable
End Function
Screenshot
