Hi salam88du,
If you don't want to use Typed DataSet, use custom object collection to populate the Crystal Report.
Refer below sample.
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
First, add an XML file in your project of your required columns which will be used to design report with .NET object.
<Customers>
<CustomerId>ALFKI</CustomerId>
<ContactName>Maria Anders</ContactName>
<City>Berlin</City>
<country>Germany</country>
</Customers>
Use the following query to generate the XML.
SELECT TOP 1 CustomerId,ContactName,City,Country
FROM Customers
FOR XML AUTO,Elements
Now, add Crystal Report to the project.
Then, select Using the Report Wizard option and click Ok.
The from the Standard Report Creation Wizard select .Net Objects and select the Object and click Finish.

Then, in the Field Explorer expand Database Fields and drag and drop in the report.

Project Structure

Class Property
public class Customer
{
public string CustomerId { get; set; }
public string Name { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
HTML
<%@ Register Assembly="CrystalDecisions.Web, Version=13.0.4000.0, Culture=neutral, PublicKeyToken=692fbea5521e1304" Namespace="CrystalDecisions.Web" TagPrefix="CR" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src='<%=ResolveUrl("~/crystalreportviewers13/js/crviewer/crv.js")%>' type="text/javascript"></script>
</head>
<body>
<form id="form1" runat="server">
<div>
<CR:CrystalReportViewer ID="CrystalReportViewer1" runat="server" ToolPanelView="None" />
</div>
</form>
</body>
</html>
Namespaces
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
using CrystalDecisions.CrystalReports.Engine;
Code
protected void Page_Load(object sender, EventArgs e)
{
ReportDocument report = new ReportDocument();
report.Load(Server.MapPath("~/CustomerReport.rpt"));
report.SetDataSource(this.GetCustomers());
CrystalReportViewer1.ReportSource = report;
}
public List<Customer> GetCustomers()
{
List<Customer> customers = new List<Customer>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT TOP 10 CustomerId,ContactName,City,Country FROM Customers", con))
{
cmd.CommandType = CommandType.Text;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
customers.Add(new Customer
{
CustomerId = sdr["CustomerId"].ToString(),
Name = sdr["ContactName"].ToString(),
City = sdr["City"].ToString(),
Country = sdr["Country"].ToString()
});
}
con.Close();
}
}
return customers;
}
Screenshot
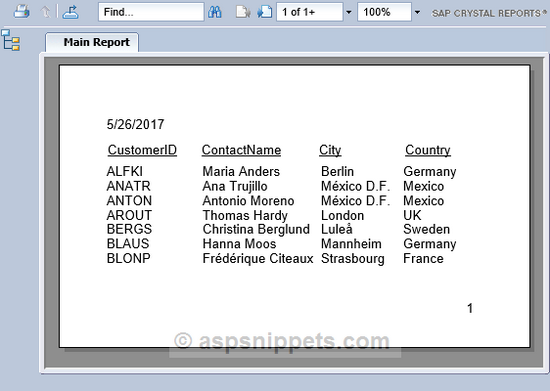
Note: You might get following error. So refer below article.