Hi mostafasalama145,
You can use convert DIV to PDF without using iTextSharp in the following ways.
1. Using SelectPdf package.
2. Using html2canvas and pdfmake plugins of JavaScript.
1. Using SelectPdf package.
HTML
<div id="divDetails" runat="server">
<h1 style="color:red;">Apple</h1>
<h3>Apple is Red.</h3>
</div>
<asp:Button ID="btnConvert" runat="server" Text="Convert" OnClick="OnConvert" />
Code
protected void OnConvert(object sender, EventArgs e)
{
//Fetch DIV content
string content = divDetails.InnerHtml;
HtmlToPdf convertToPDF = new HtmlToPdf();
PdfDocument pdfDoc = new PdfDocument();
//Converts HTML string to the PDF
pdfDoc = convertToPDF.ConvertHtmlString(content);
//Determine the BYTE array of Generated PDF
byte[] bytes = pdfDoc.Save();
//Read SMTP section from Web.Config.
SmtpSection smtpSection = (SmtpSection)ConfigurationManager.GetSection("system.net/mailSettings/smtp");
string host = smtpSection.Network.Host;
int port = smtpSection.Network.Port;
using (MimeMessage mm = new MimeMessage())
{
mm.From.Add(new MailboxAddress("Sender", "sender@gmail.com"));
mm.To.Add(new MailboxAddress("Recepient", "sender@gmail.com"));
mm.Subject = "Convert DIV to PDF using SelectPDF library";
BodyBuilder builder = new BodyBuilder()
{
TextBody = "The DIV is sucessfully converted to PDF using SelectPDF library."
};
//Adding PDF as attachment.
builder.Attachments.Add("Converted PDF.pdf", bytes);
mm.Body = builder.ToMessageBody();
using (SmtpClient smtp = new SmtpClient())
{
//Set to False to avoid Certificate verification.
smtp.CheckCertificateRevocation = false;
smtp.Connect(host, port, SecureSocketOptions.Auto);
smtp.Authenticate("sender@gmail.com", "<Password>");
smtp.Send(mm);
smtp.Disconnect(true);
}
}
ClientScript.RegisterStartupScript(this.GetType(), "alert", "alert('Email sent.');", true);
}
Output

2. Using html2canvas and pdfmake plugins of JavaScript
HTML
In this case, ScriptManager is used to enable Page method as Web Method will be called to send email.
<form runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server" EnablePageMethods="true">
</asp:ScriptManager>
</form>
<div id="divDetails" runat="server">
<h1 style="color: red;">Apple</h1>
<h3>Apple is Red.</h3>
</div>
<input type="button" id="btnExport" value="Export" onclick="return Export()" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.2.7/pdfmake.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/0.4.1/html2canvas.min.js"></script>
<script type="text/javascript">
function Export() {
html2canvas(document.getElementById('divDetails'), {
onrendered: function (canvas) {
var data = canvas.toDataURL();
var docDefinition = {
content: [{
image: data,
width: 500
}]
};
//Creates PDF
var pdfDocGenerator = pdfMake.createPdf(docDefinition);
//Determine BASE64 string
pdfDocGenerator.getBase64(function (base64) {
SendByteArray(base64);
});
}
});
}
//Calls Server-Side Web Method
function SendByteArray(base64) {
PageMethods.SendEmail(base64, OnSuccess);
}
function OnSuccess(response) {
alert(response);
}
</script>
Code (Web Method)
[WebMethod]
public static string SendEmail(string bytes)
{
//Determine the BYTE array of Generated PDF
byte[] pdfByte = Convert.FromBase64String(bytes);
//Read SMTP section from Web.Config.
SmtpSection smtpSection = (SmtpSection)ConfigurationManager.GetSection("system.net/mailSettings/smtp");
string host = smtpSection.Network.Host;
int port = smtpSection.Network.Port;
using (MimeMessage mm = new MimeMessage())
{
mm.From.Add(new MailboxAddress("Sender", "sender@gmail.com"));
mm.To.Add(new MailboxAddress("Recepient", "sender@gmail.com"));
mm.Subject = "Convert DIV to PDF using html2canvas and pdfmake plugins";
BodyBuilder builder = new BodyBuilder()
{
TextBody = "The DIV is sucessfully converted using html2canvas and pdfmake plugins."
};
//Adding PDF as attachment.
builder.Attachments.Add("Converted PDF.pdf", pdfByte);
mm.Body = builder.ToMessageBody();
using (SmtpClient smtp = new SmtpClient())
{
//Set to False to avoid Certificate verification.
smtp.CheckCertificateRevocation = false;
smtp.Connect(host, port, SecureSocketOptions.Auto);
smtp.Authenticate("sender@gmail.com", "<Password>");
smtp.Send(mm);
smtp.Disconnect(true);
}
}
return "Email sent.";
}
Screenshot
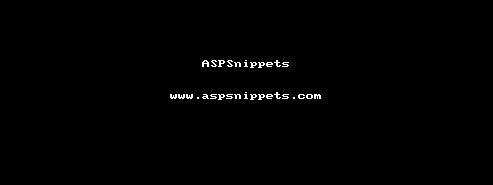
Downloads
Download Sample