Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used table named Fruits whose schema is defined as follows.
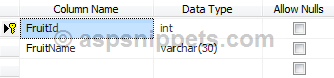
The Fruits table has the following records.
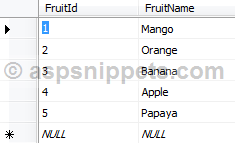
You can download the database table SQL by clicking the download link below.
Download SQL file
For reading connectrion string refer below article.
Model
Fruit
public class Fruit
{
public int FruitId { get; set; }
public string FruitName { get; set; }
}
FruitModel
public class FruitModel
{
public List<SelectListItem> Fruits { get; set; }
public int[] FruitIds { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
FruitModel model = new FruitModel();
model.Fruits = this.Context.Fruits
.Select(x => new SelectListItem
{
Text = x.FruitName,
Value = Convert.ToString(x.FruitId)
}).ToList();
return View(model);
}
[HttpPost]
public IActionResult Index(FruitModel fruit)
{
fruit.Fruits = this.Context.Fruits
.Select(x => new SelectListItem
{
Text = x.FruitName,
Value = Convert.ToString(x.FruitId)
}).ToList();
if (fruit.FruitIds != null)
{
List<SelectListItem> selectedItems = fruit.Fruits
.Where(p => fruit.FruitIds.Contains(int.Parse(p.Value))).ToList();
ViewBag.Message = "Selected Fruits:";
foreach (var selectedItem in selectedItems)
{
selectedItem.Selected = true;
ViewBag.Message += "\\n" + selectedItem.Text;
}
}
return View(fruit);
}
}
View
@model Core_MVC_AutoComplete.Models.FruitModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/css/bootstrap.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/js/bootstrap.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.rawgit.com/davidstutz/bootstrap-multiselect/master/dist/css/bootstrap-multiselect.css" />
<script type="text/javascript" src="https://cdn.rawgit.com/davidstutz/bootstrap-multiselect/master/dist/js/bootstrap-multiselect.js"></script>
<script type="text/javascript">
$(function () {
$('.listbox').multiselect({
includeSelectAllOption: true
});
});
</script>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
@Html.Label("Fruits:")
<br /><br />
@Html.ListBoxFor(m => m.FruitIds, Model.Fruits, new { @class = "listbox" })
<br /><br />
<input type="submit" value="Submit" />
}
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
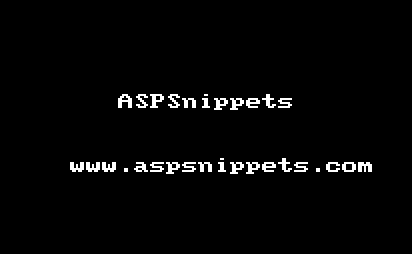