Hi Mohammadmk,
Refering the below articles i have created the example.
Client Side Paging in WebGrid in ASP.Net MVC
ASP.Net MVC: Call Stored Procedure using Entity Framework
Check this example. Now please take its reference and correct your code.
Database
I am making use of Microsoft's Northwind Database. You can download it from here.
Download and install Northwind Database
Stored Procedure
CREATE PROCEDURE [dbo].[Customers_GetCustomersPageWise]
@PageIndex INT = 1,
@PageSize INT = 20,
@RecordCount INT OUTPUT
AS
BEGIN
SET NOCOUNT ON;
SELECT (ROW_NUMBER() OVER(Order By CustomerId)) AS RowNumber,
[CustomerID]
,[CompanyName]
,[ContactName]
,[ContactTitle]
,[Address]
,[City]
,[Region]
,[PostalCode]
,[Country]
,[Phone]
,[Fax]
INTO #Results
FROM [Customers]
SELECT @RecordCount = Count(*) FROM #Results
SELECT * FROM #Results WHERE
RowNumber BETWEEN (@PageIndex-1)*@PageSize + 1 AND (((@PageIndex-1)*@PageSize + 1)+@PageSize)-1 OR @PageIndex = -1
DROP TABLE #Results
END
In Add Function Import dialog you need to specify the Function Import Name which is the name of the function used to call the Stored Procedure and then select the Stored Procedure that will be executed when the function is called.
The Return Type is selected as Entities which is the Customer Entity class.

Model
public class CustomerModel
{
public List<Customer> Customers { get; set; }
public int PageIndex { get; set; }
public int PageSize { get; set; }
public int RecordCount { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
List<Customer> dummy = new List<Customer>();
dummy.Add(new Customer());
return View(dummy);
}
[HttpPost]
public JsonResult AjaxMethod(int pageIndex)
{
int pageSize = 10;
NorthwindEntities entities = new NorthwindEntities();
// Hold the output parameter.
ObjectParameter recordCount = new ObjectParameter("RecordCount", typeof(int));
List<Customer> customers = entities.CustomersPageWise(pageIndex, pageSize, recordCount).ToList();
CustomerModel model = new CustomerModel();
model.PageIndex = pageIndex;
model.PageSize = pageSize;
model.RecordCount = Convert.ToInt32(recordCount.Value);
model.Customers = customers;
return Json(model);
}
}
View
@model IEnumerable<Customer>
@{
Layout = null;
WebGrid webGrid = new WebGrid(source: Model, canSort: false, canPage: false);
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<style type="text/css">
body {
font-family: Arial;
font-size: 10pt;
}
table {
border: 1px solid #ccc;
border-collapse: collapse;
background-color: #fff;
}
table th {
background-color: #B8DBFD;
color: #333;
font-weight: bold;
}
table th, table td {
padding: 5px;
border: 1px solid #ccc;
}
table, table table td {
border: 0px solid #ccc;
}
.Pager span {
text-align: center;
color: #333;
display: inline-block;
width: 20px;
background-color: #B8DBFD;
margin-right: 3px;
line-height: 150%;
border: 1px solid #B8DBFD;
}
.Pager a {
text-align: center;
display: inline-block;
width: 20px;
background-color: #ccc;
color: #333;
border: 1px solid #ccc;
margin-right: 3px;
line-height: 150%;
text-decoration: none;
}
</style>
</head>
<body>
<h4>Customers</h4>
<hr />
@webGrid.GetHtml(
htmlAttributes: new { @id = "WebGrid", @class = "Grid" },
columns: webGrid.Columns(
webGrid.Column("CustomerID", "CustomerID"),
webGrid.Column("ContactName", "ContactName"),
webGrid.Column("City", "City"),
webGrid.Column("Country", "Country")))
<br />
<div class="Pager"></div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script src="~/Scripts/ASPSnippets_Pager.min.js"></script>
<script type="text/javascript">
$(function () {
GetCustomers(1);
});
$("body").on("click", ".Pager .page", function () {
GetCustomers(parseInt($(this).attr('page')));
});
function GetCustomers(pageIndex) {
$.ajax({
type: "POST",
url: "/Home/AjaxMethod",
data: '{pageIndex: ' + pageIndex + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
failure: function (response) {
alert(response.d);
},
error: function (response) {
alert(response.d);
}
});
};
function OnSuccess(response) {
var model = response;
var row = $("#WebGrid tbody tr:last-child").clone(true);
$("#WebGrid tbody tr").remove();
$.each(model.Customers, function () {
var customer = this;
$("td", row).eq(0).html(customer.CustomerID);
$("td", row).eq(1).html(customer.ContactName);
$("td", row).eq(2).html(customer.City);
$("td", row).eq(3).html(customer.Country);
$("#WebGrid").append(row);
row = $("#WebGrid tbody tr:last-child").clone(true);
});
$(".Pager").ASPSnippets_Pager({
ActiveCssClass: "current",
PagerCssClass: "pager",
PageIndex: model.PageIndex,
PageSize: model.PageSize,
RecordCount: model.RecordCount
});
};
</script>
</body>
</html>
Screenshot
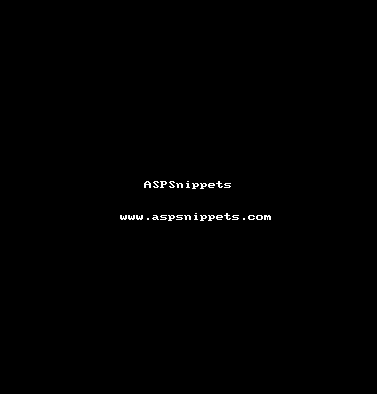