Hi indradeo,
Please refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
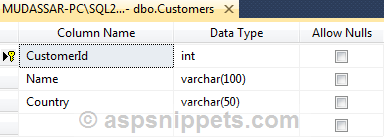
I have already inserted few records in the table.
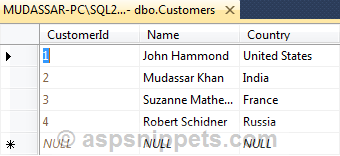
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="CustomerId" HeaderText="CustomerId" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<asp:Label ID="lblCountry" runat="server" Text='<%#Eval("Country") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Action">
<ItemTemplate>
<asp:LinkButton ID="lnkEdit" runat="server" Text="Edit"></asp:LinkButton>
<asp:LinkButton ID="lnkDelete" runat="server" Text="Delete"></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Namespaces
C#
using System.Configuration;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if(!this.IsPostBack)
{
this.BindGridView();
foreach (GridViewRow row in gvCustomers.Rows)
{
(row.FindControl("lnkEdit") as LinkButton).Visible = false;
}
}
}
private void BindGridView()
{
string ConString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(ConString))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Customers", con))
{
con.Open();;
gvCustomers.DataSource = cmd.ExecuteReader();
gvCustomers.DataBind();
con.Close();
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGridView()
For Each row As GridViewRow In gvCustomers.Rows
TryCast(row.FindControl("lnkEdit"), LinkButton).Visible = False
Next
End If
End Sub
Private Sub BindGridView()
Dim ConString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(ConString)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM Customers", con)
con.Open()
gvCustomers.DataSource = cmd.ExecuteReader()
gvCustomers.DataBind()
con.Close()
End Using
End Using
End Sub
Screenshot
