Hi ramco1917,
I have checked its working.
You need to make sure you have set align property "center" and verticalAlign property "top".
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
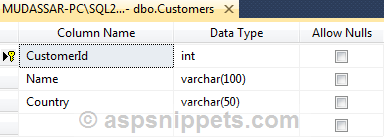
I have already inserted few records in the table.
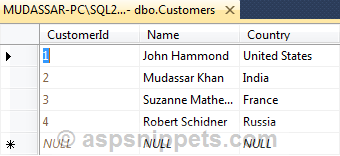
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
public int CustomerID { get; set; }
public string Name { get; set; }
public string Country { get; set; }
public bool IsActive { get; set; }
public List<Customer> Customers { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
using (CustomerEntities entities = new CustomerEntities())
{
CustomerModel model = new CustomerModel();
model.Customers = entities.Customers.ToList();
return View(model);
}
}
[HttpPost]
public ActionResult Index(CustomerModel model, string hfAU)
{
using (CustomerEntities entities = new CustomerEntities())
{
if (hfAU == "U")
{
Customer customerUpdate = entities.Customers.Where(x => x.CustomerId == model.CustomerID).FirstOrDefault();
customerUpdate.Name = model.Name;
customerUpdate.Country = model.Country;
entities.SaveChanges();
TempData["Message"] = " Customer updated successfully.";
}
if (hfAU == "A")
{
entities.Customers.Add(new Customer
{
Name = model.Name,
Country = model.Country
});
entities.SaveChanges();
TempData["Message"] = " Customer inserted successfully.";
}
return RedirectToAction("Index");
}
}
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult DeleteCustomer(int id)
{
using (CustomerEntities entities = new CustomerEntities())
{
Customer customer = entities.Customers.Where(x => x.CustomerId == id).FirstOrDefault();
entities.Customers.Remove(customer);
entities.SaveChanges();
TempData["Message"] = " Customer deleted successfully.";
return RedirectToAction("Index");
}
}
}
View
@model Notification_Popup_MVC.Models.CustomerModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body class="container">
<div class="table-title">
<div class="row">
<div class="col-sm-6 text-right">
<button id="btnAdd" type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal">
<i class="fa fa-plus"></i> Add New
</button>
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12">
<div class="box">
<div class="box-body">
<table class="table" id="tblCustomers">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
<th>IsActive</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model.Customers)
{
var active = item.Country == "India" ? true : false;
<tr>
<td>@Html.DisplayFor(modelItem => item.CustomerId)</td>
<td>@Html.DisplayFor(modelItem => item.Name)</td>
<td>@Html.DisplayFor(modelItem => item.Country)</td>
<td>@Html.DisplayFor(modelItem => active)</td>
<td>
<a class='btn btn-primary btn-sm' id='btnEdit'><i class='fa fa-pencil'></i> Edit</a>
<a id="btnDelete" class="btn btn-danger btn-sm" data-toggle="modal"
data-target="#DeleteModal-@item.CustomerId" style='margin-left:5px'><i class='fa fa-trash'></i> Delete</a>
@using (Html.BeginForm("DeleteCustomer", "Home", new { id = item.CustomerId }, FormMethod.Post, null))
{
@Html.AntiForgeryToken()
<div class="modal" tabindex="-1" role="dialog" id="DeleteModal-@item.CustomerId">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Delete Confirmation</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<p>Are you sure you want to delete this record ?</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Cancel</button>
<button type="submit" class="btn btn-danger">Delete</button>
</div>
</div>
</div>
</div>
}
</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
</div>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
<input type="hidden" id="hfAU" name="hfAU" />
<div id="myModal" class="modal" tabindex="-1" role="dialog">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Customer Details Form</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="form-group row">
<input type="hidden" id="hfId" name="CustomerID" />
<label>Name:</label>
<input type="text" id="txtName" name="Name" value="" class="form-control" />
</div>
<div class="form-group row">
<label>Country:</label>
<input type="text" id="txtCountry" name="Country" value="" class="form-control" />
</div>
<div class="form-group row">
<label for="IsActive" class="col-sm-2 col-form-label">IsActive</label>
<div class="form-check col-sm-10">
<input class="form-check-input" type="checkbox" value="true" id="IsActive">
<input type="hidden" id="hfIsActive" name="IsActive" />
</div>
</div>
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-primary">Save changes</button>
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
}
<script type="text/javascript" src="https://code.jquery.com/jquery-3.2.1.slim.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.20/js/jquery.dataTables.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.20/css/jquery.dataTables.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" />
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<script type="text/javascript" src="https://www.jqueryscript.net/demo/Simple-Flexible-jQuery-Alert-Notification-Plugin-notify-js/js/notify.js"></script>
<link rel="stylesheet" href="https://www.jqueryscript.net/demo/Simple-Flexible-jQuery-Alert-Notification-Plugin-notify-js/css/notify.css" />
<script type="text/javascript">
$(function () {
$("#tblCustomers").dataTable({
order: [],
columnDefs: [{ orderable: false, targets: [3, 4] }],
});
$('body').on('click', '[id*=btnEdit]', function () {
$('#hfAU').val('U');
$('#IsActive').removeAttr('checked').removeAttr("disabled");
$('#hfIsActive').val('false');
var data = $(this).closest('tr').find('td');
var id = data.eq(0).html();
var name = data.eq(1).html();
var country = data.eq(2).html();
var isActive = $(this).closest('tr').find('input[type=checkbox]').is(':checked');
$('#hfId').val(id);
$('#txtName').val(name);
$('#txtCountry').val(country);
if (isActive) {
$('#IsActive').attr('checked', 'checked');
$('#hfIsActive').val('true');
}
$('#myModal').modal('show');
});
$('#IsActive').click(function () {
if ($(this).is(':checked')) {
$('#hfIsActive').val('true');
} else {
$('#hfIsActive').val('false');
}
});
$('body').on('click', '[id*=btnAdd]', function () {
$('#hfId').val('');
$('#txtName').val('');
$('#txtCountry').val('');
$('#hfAU').val('A');
$('#IsActive').attr('checked', 'checked').attr("disabled", true);
});
});
</script>
@if (TempData["Message"] != null)
{
<script type="text/javascript">
window.onload = function () {
var message = "@TempData["Message"]";
$.notify(message, {
type: 'success',
icon: 'check',
align: "center",
verticalAlign: "top",
animation: true,
animationType: "drop",
close: true
});
};
</script>
}
</body>
</html>
Screenshot
