Hi LessClue,
Refer the below sample code for your reference and implement it in your code as per your logic.
First read the CSV to DataTable and then filter using multiple condition.
CSV File
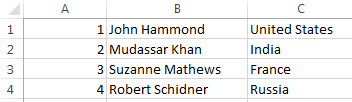
HTML
Id: <asp:TextBox ID="txtId" runat="server"></asp:TextBox><br />
Name: <asp:TextBox ID="txtName" runat="server"></asp:TextBox><br />
Country: <asp:TextBox ID="txtCountry" runat="server"></asp:TextBox><br />
<asp:Button ID="btnSearch" runat="server" Text="Search" OnClick="OnSearch" />
<hr />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
Namespaces
C#
using System.Data;
VB.Net
Imports System.Data
Code
C#
protected void OnSearch(object sender, EventArgs e)
{
string csvPath = Server.MapPath("~/Files/Sample.csv");
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[3] {
new DataColumn("Id", typeof(string)),
new DataColumn("Name", typeof(string)),
new DataColumn("Country",typeof(string)) });
string csvData = System.IO.File.ReadAllText(csvPath);
foreach (string row in csvData.Split('\n'))
{
if (!string.IsNullOrEmpty(row))
{
dt.Rows.Add();
int i = 0;
foreach (string cell in row.Split(','))
{
dt.Rows[dt.Rows.Count - 1][i] = cell;
i++;
}
}
}
IEnumerable<DataRow> result = (from row in dt.AsEnumerable()
where (row["Id"].ToString() == txtId.Text.Trim() || string.IsNullOrEmpty(txtId.Text.Trim()))
&& (row["Name"].ToString().ToLower().Contains(txtName.Text.Trim().ToLower()) || string.IsNullOrEmpty(txtName.Text.Trim()))
&& (row["Country"].ToString().ToLower().StartsWith(txtCountry.Text.Trim().ToLower()) || string.IsNullOrEmpty(txtCountry.Text.Trim()))
select row).ToList();
DataTable final = dt.Clone();
if (result.Count() > 0)
{
final = result.CopyToDataTable();
}
GridView1.DataSource = final;
GridView1.DataBind();
}
VB.Net
Protected Sub OnSearch(ByVal sender As Object, ByVal e As EventArgs)
Dim csvPath As String = Server.MapPath("~/Files/Sample.csv")
Dim dt As DataTable = New DataTable()
dt.Columns.AddRange(New DataColumn(2) {
New DataColumn("Id", GetType(Integer)),
New DataColumn("Name", GetType(String)),
New DataColumn("Country", GetType(String))})
Dim csvData As String = System.IO.File.ReadAllText(csvPath)
For Each row As String In csvData.Split(vbLf)
If Not String.IsNullOrEmpty(row) Then
dt.Rows.Add()
Dim i As Integer = 0
For Each cell As String In row.Split(","c)
dt.Rows(dt.Rows.Count - 1)(i) = cell
i += 1
Next
End If
Next
Dim result As IEnumerable(Of DataRow) = (From row In dt.AsEnumerable()
Where (row("Id").ToString() = txtId.Text.Trim() OrElse String.IsNullOrEmpty(txtId.Text.Trim())) _
AndAlso (row("Name").ToString().ToLower().Contains(txtName.Text.Trim().ToLower()) OrElse String.IsNullOrEmpty(txtName.Text.Trim())) _
AndAlso (row("Country").ToString().ToLower().StartsWith(txtCountry.Text.Trim().ToLower()) OrElse String.IsNullOrEmpty(txtCountry.Text.Trim()))
Select row).ToList()
Dim final As DataTable = dt.Clone()
If result.Count() > 0 Then
final = result.CopyToDataTable()
End If
GridView1.DataSource = final
GridView1.DataBind()
End Sub