Hi aakhan314,
After populating the Chart you need to convert the Chart as Image.
Then use the Image to print using Javascript. It will work in all browser.
Using the below article i have created the example.
Populate Bar and Column Charts from database using ASP.Net Chart control
Refer the below sample.
HTML
<div id="cChart">
<asp:Chart ID="grChart" runat="server" Height="300px" Width="400px">
<Series>
<asp:Series Name="Series1" Legend="Legend1">
</asp:Series>
</Series>
<ChartAreas>
<asp:ChartArea Name="ChartArea1">
</asp:ChartArea>
</ChartAreas>
<Legends>
<asp:Legend Name="Legend1">
</asp:Legend>
</Legends>
</asp:Chart>
</div>
<input type="button" value="Print" onclick="printChart()" style="width: 99px; height: 26px;" />
<script type="text/javascript">
function printChart() {
// Get Chart Image in BASE64 String format.
var chartImageUrl = '<%=this.ChartImage%>';
var html = '<html>\n<head>\n';
html += '<title></title>\n';
html += '<link rel="stylesheet" type="text/css" href="print.css" media="print" />\n';
html += '\n</head>\n<body>\n';
html += '\n<img src="' + chartImageUrl + '">';
html += '\n</body>\n</html>';
var printWin = window.open('', '_blank');
printWin.document.open();
printWin.document.write(html);
printWin.document.close();
printWin.print();
}
</script>
Namespaces
C#
using System.IO;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Web.UI.DataVisualization.Charting;
VB.Net
Imports System.IO
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Web.UI.DataVisualization.Charting
Code
C#
protected string ChartImage { get; set; }
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
string query = "SELECT ShipCity, COUNT(OrderId) [Total] FROM Orders WHERE ShipCountry = 'France' GROUP BY ShipCity";
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlDataAdapter sda = new SqlDataAdapter(query, con))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
grChart.DataSource = dt;
grChart.Series[0].ChartType = SeriesChartType.Bar;
grChart.Legends[0].Enabled = false;
grChart.Series[0].XValueMember = "ShipCity";
grChart.Series[0].YValueMembers = "Total";
grChart.DataBind();
// Convert the Chart control to BASE64 String format.
this.ChartImage = "data:image/png;base64," + this.GetChartBase64Image(grChart);
}
}
}
}
}
private string GetChartBase64Image(Chart chart)
{
using (MemoryStream memStream = new MemoryStream())
{
chart.SaveImage(memStream, ChartImageFormat.Png);
byte[] imageArray = memStream.ToArray();
return Convert.ToBase64String(imageArray);
}
}
VB.Net
Protected Property ChartImage As String
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim query As String = "SELECT ShipCity, COUNT(OrderId) [Total] FROM Orders WHERE ShipCountry = 'France' GROUP BY ShipCity"
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constr)
Using sda As SqlDataAdapter = New SqlDataAdapter(query, con)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
grChart.DataSource = dt
grChart.Series(0).ChartType = SeriesChartType.Bar
grChart.Legends(0).Enabled = False
grChart.Series(0).XValueMember = "ShipCity"
grChart.Series(0).YValueMembers = "Total"
grChart.DataBind()
' Convert the Chart control to BASE64 String format.
Me.ChartImage = "data:image/png;base64," & Me.GetChartBase64Image(grChart)
End Using
End Using
End Using
End If
End Sub
Private Function GetChartBase64Image(chart As Chart) As String
Using memStream As MemoryStream = New MemoryStream()
chart.SaveImage(memStream, ChartImageFormat.Png)
Dim imageArray As Byte() = memStream.ToArray()
Return Convert.ToBase64String(imageArray)
End Using
End Function
Screenshot
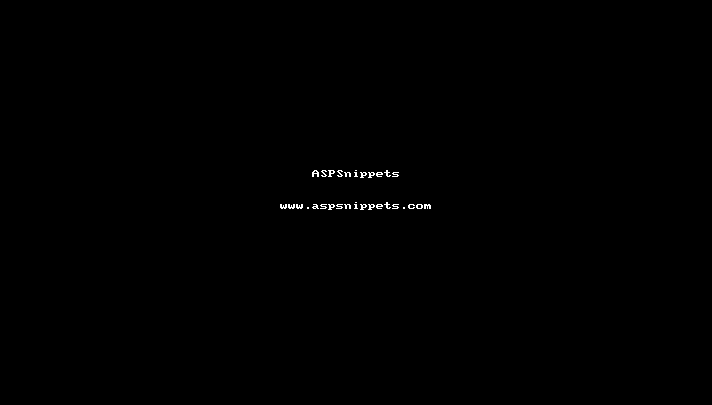
Downloads
Download Sample