Hi Poonam123,
Refer below sample.
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
HTML
<table class="Grid" cellspacing="0" rules="all" border="1" id="Table1" style="width: 500px;
border-collapse: collapse;">
<tr>
<th scope="col" style="width: 200px;">
Customer Name
</th>
<th scope="col" style="width: 100px;">
City
</th>
<th scope="col" style="width: 100px;">
Country
</th>
<th scope="col" style="width: 100px;">
Postal Code
</th>
</tr>
</table>
<div id="dvGrid" style="height: 250px; overflow: auto; width: 517px">
<asp:GridView ID="gvCustomers" runat="server" AutoGenerateColumns="false" CssClass="Grid"
Width="500">
<Columns>
<asp:BoundField DataField="ContactName" HeaderText="Customer Name" ItemStyle-CssClass="name"
ItemStyle-Width="200" HeaderStyle-Width="200" />
<asp:BoundField DataField="City" HeaderText="City" ItemStyle-CssClass="city" ItemStyle-Width="100"
HeaderStyle-Width="100" />
<asp:BoundField DataField="Country" HeaderText="Country" ItemStyle-CssClass="country"
ItemStyle-Width="100" HeaderStyle-Width="100" />
<asp:BoundField DataField="PostalCode" HeaderText="Postal Code" ItemStyle-CssClass="postal"
ItemStyle-Width="100" HeaderStyle-Width="100" />
</Columns>
</asp:GridView>
</div>
<script src="jquery.min.js" type="text/javascript"></script>
<script type="text/javascript">
var pageIndex = 1;
var pageCount = <%=this.PageCount %>;
$(function () {
$("[id$=gvCustomers] tr").eq(0).remove();
});
$("#dvGrid").on("scroll", function (e) {
var $o = $(e.currentTarget);
if ($o[0].scrollHeight - $o.scrollTop() <= $o.outerHeight()) {
GetRecords();
}
});
function GetRecords() {
pageIndex++;
if (pageIndex == 2 || pageIndex <= pageCount) {
if ($("[id$=gvCustomers] .loader").length == 0) {
var row = $("[id$=gvCustomers] tr").eq(0).clone(true);
row.addClass("loader");
row.children().remove();
row.append('<td colspan = "999" style = "background-color:white"><img id="loader" alt="" src="103.gif" /></td>');
$("[id$=gvCustomers]").append(row);
}
$.ajax({
type: "POST",
url: "Default.aspx/GetCustomers",
data: '{pageIndex: ' + pageIndex + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
error: function (response) {
alert(response.d);
}
});
} else {
return false;
}
}
function OnSuccess(response) {
var xmlDoc = $.parseXML(response.d);
var xml = $(xmlDoc);
var customers = xml.find("Table1");
$("[id$=gvCustomers] .loader").remove();
customers.each(function () {
var customer = $(this);
var row = $("[id$=gvCustomers] tr").eq(0).clone(true);
$(".name", row).html(customer.find("ContactName").text());
$(".city", row).html(customer.find("City").text());
$(".postal", row).html(customer.find("PostalCode").text());
$(".country", row).html(customer.find("Country").text());
$("[id$=gvCustomers]").append(row);
});
$("#loader").hide();
}
</script>
Namespaces
C#
using System.Data;
using System.Web.Services;
using System.Configuration;
using System.Data.SqlClient;
using System.Linq;
VB.Net
Imports System.Data
Imports System.Web.Services
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Linq
Code
C#
protected int PageCount { get; set; }
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
DataSet ds = new DataSet();
int count = GetCustomersPageWise().Tables[0].Rows.Count / 10;
int mod = GetCustomersPageWise().Tables[0].Rows.Count % 10;
if (mod > 0)
{
PageCount = count + 1;
}
else
{
PageCount = count;
}
ds.Tables.Add(GetCustomersPageWise().Tables[0].AsEnumerable().Skip(0).Take(10).CopyToDataTable());
gvCustomers.DataSource = ds;
gvCustomers.DataBind();
}
}
public static DataSet GetCustomersPageWise()
{
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("SELECT * FROM Customers ORDER BY CustomerID", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataSet ds = new DataSet())
{
sda.Fill(ds, "Customers");
HttpContext.Current.Session["ds"] = ds;
return ds;
}
}
}
}
}
[WebMethod]
public static string GetCustomers(int pageIndex)
{
DataSet ds = HttpContext.Current.Session["ds"] as DataSet;
DataSet dsResult = new DataSet();
int startIndex = (pageIndex - 1) * 10;
if ((ds.Tables[0].AsEnumerable().Skip(startIndex)).Count() > 0)
{
dsResult.Tables.Add(ds.Tables[0].AsEnumerable().Skip(startIndex).Take(10).CopyToDataTable());
}
return dsResult.GetXml();
}
VB.Net
Protected Property PageCount As Integer
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Dim ds As DataSet = New DataSet()
Dim count As Integer = GetCustomersPageWise().Tables(0).Rows.Count / 10
Dim [mod] As Integer = GetCustomersPageWise().Tables(0).Rows.Count Mod 10
If [mod] > 0 Then
PageCount = count + 1
Else
PageCount = count
End If
ds.Tables.Add(GetCustomersPageWise().Tables(0).AsEnumerable().Skip(0).Take(10).CopyToDataTable())
gvCustomers.DataSource = ds
gvCustomers.DataBind()
End If
End Sub
Public Shared Function GetCustomersPageWise() As DataSet
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("SELECT * FROM Customers ORDER BY CustomerID", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using ds As DataSet = New DataSet()
sda.Fill(ds, "Customers")
HttpContext.Current.Session("ds") = ds
Return ds
End Using
End Using
End Using
End Using
End Function
<WebMethod()>
Public Shared Function GetCustomers(ByVal pageIndex As Integer) As String
Dim ds As DataSet = TryCast(HttpContext.Current.Session("ds"), DataSet)
Dim dsResult As DataSet = New DataSet()
Dim startIndex As Integer = (pageIndex - 1) * 10
If (ds.Tables(0).AsEnumerable().Skip(startIndex).Count() > 0) Then
dsResult.Tables.Add(ds.Tables(0).AsEnumerable().Skip(startIndex).Take(10).CopyToDataTable())
End If
Return dsResult.GetXml()
End Function
Screenshot
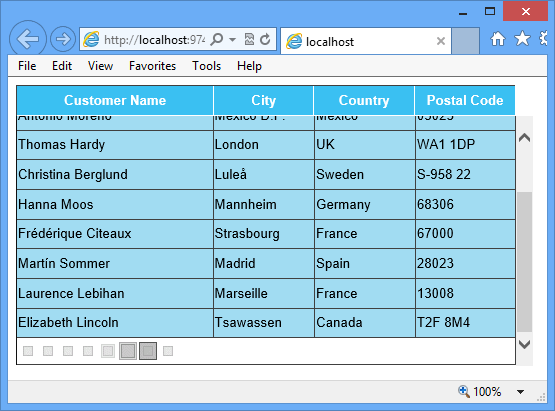
Note:
var pageCount = <%=this.PageCount %>;
Change the above line with below for VB.Net.
var pageCount = <%=Me.PageCount %>;