Hi akhter,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table tblFiles with the schema as follows.
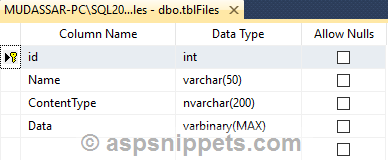
I have already inserted few record in the table.
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
C#
<asp:Repeater ID="rptImages" runat="server">
<HeaderTemplate>
<table>
<thead>
<tr>
<th>Name</th>
<th>Photo</th>
</tr>
</thead>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td><%# Eval("Name") %></td>
<td>
<img src='data:image/jpg;base64,<%# !string.IsNullOrEmpty(Eval("Name").ToString()) ? Convert.ToBase64String((byte[])Eval("Data")) : string.Empty %>'
alt="image" height="100" width="100" style="border: 5px solid black" />
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
VB.Net
<asp:Repeater ID="rptImages" runat="server">
<HeaderTemplate>
<table>
<thead>
<tr>
<th>Name</th>
<th>Photo</th>
</tr>
</thead>
</HeaderTemplate>
<ItemTemplate>
<tr>
<td><%# Eval("Name") %></td>
<td>
<img src='data:image/jpg;base64,<%# If(Not String.IsNullOrEmpty(Eval("Name")), Convert.ToBase64String(CType(Eval("Data"), Byte())), String.Empty) %>'
alt="image" height="100" width="100" style="border: 5px solid black" />
</td>
</tr>
</ItemTemplate>
<FooterTemplate>
</table>
</FooterTemplate>
</asp:Repeater>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT TOP 3 * FROM tblFiles";
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
sda.SelectCommand = cmd;
DataTable dt = new DataTable();
sda.Fill(dt);
rptImages.DataSource = dt;
rptImages.DataBind();
}
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "SELECT TOP 3 * FROM tblFiles"
Dim cmd As SqlCommand = New SqlCommand(query)
Using con As SqlConnection = New SqlConnection(conString)
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.Connection = con
sda.SelectCommand = cmd
Dim dt As DataTable = New DataTable()
sda.Fill(dt)
rptImages.DataSource = dt
rptImages.DataBind()
End Using
End Using
End If
End SubProtected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "SELECT TOP 3 * FROM tblFiles WHERE ContentType = 'image/jpeg'"
Dim cmd As SqlCommand = New SqlCommand(query)
Using con As SqlConnection = New SqlConnection(conString)
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.Connection = con
sda.SelectCommand = cmd
Dim dt As DataTable = New DataTable()
sda.Fill(dt)
rptImages.DataSource = dt
rptImages.DataBind()
End Using
End Using
End If
End Sub
Screenshot
