Hi jovceka,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
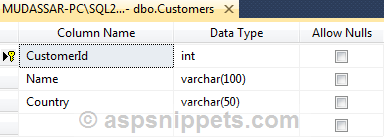
I have already inserted few records in the table.
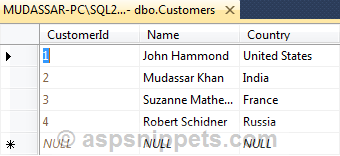
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<table id="example">
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
</tr>
</table>
<br />
<br />
<table>
<tr>
<td>Name</td>
<td><input type="text" id="txtName" /></td>
</tr>
<tr>
<td>Country</td>
<td><input type="text" id="txtCountry" /></td>
</tr>
<tr>
<td colspan="2"><input type="button" value="Insert" id="btnAdd" /></td>
</tr>
</table>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
GetProduct();
$('#btnAdd').click(function () {
var obj = {};
obj.Name = $('#txtName').val();
obj.Country = $('#txtCountry').val();
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
url: "WebService.asmx/AddCustomer",
dataType: "json",
data: JSON.stringify(obj),
success: function (data) {
if (data.d == 'true') {
GetProduct();
}
else {
$('#txtName').val() = "";
$('#txtCountry').val() = "";
}
},
error: function (result) {
alert("Error");
}
});
})
});
function GetProduct() {
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
url: "WebService.asmx/GetData",
data: {},
dataType: "json",
success: function (data) {
var table = $('#example');
$(table).find("tr:gt(0)").remove();
var rows = "";
for (var i = 0; i < data.d.length; i++) {
var id = data.d[i].Id;
var name = data.d[i].Name;
var country = data.d[i].Country;
rows += "<tr><td>" + id + "</td><td>" + name + "</td><td>" + country + "</td></tr>";
}
table.append(rows);
},
error: function (response) {
alert("Error while Showing update data");
}
});
}
</script>
Namespaces
C#
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Services;
VB.Net
Imports System
Imports System.Collections.Generic
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.Web.Services
WebService
C#
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class WebService : System.Web.Services.WebService
{
[WebMethod]
public List<Customer> GetData()
{
List<Customer> customers = new List<Customer>();
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT CustomerId,Name,Country FROM Customers";
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
cmd.Connection = con;
con.Open();
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
customers.Add(new Customer
{
Id = Convert.ToInt32(sdr["CustomerId"]),
Name = sdr["Name"].ToString(),
Country = sdr["Country"].ToString()
});
}
con.Close();
}
return customers;
}
[WebMethod]
public string AddCustomer(string Name, string Country)
{
string status = "";
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "INSERT INTO Customers VALUES(@Name,@Country)";
using (SqlConnection con = new SqlConnection(conString))
{
SqlCommand cmd = new SqlCommand(query);
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Name", Name);
cmd.Parameters.AddWithValue("@Country", Country);
con.Open();
status = cmd.ExecuteNonQuery() > 0 ? "true" : "false";
con.Close();
}
return status;
}
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
VB.Net
<WebService([Namespace]:="http://tempuri.org/")>
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)>
<System.Web.Script.Services.ScriptService>
Public Class WebService
Inherits System.Web.Services.WebService
<WebMethod>
Public Function GetData() As List(Of Customer)
Dim customers As List(Of Customer) = New List(Of Customer)()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "SELECT CustomerId,Name,Country FROM Customers"
Dim cmd As SqlCommand = New SqlCommand(query)
Using con As SqlConnection = New SqlConnection(conString)
cmd.Connection = con
con.Open()
Dim sdr As SqlDataReader = cmd.ExecuteReader()
While sdr.Read()
customers.Add(New Customer With {
.Id = Convert.ToInt32(sdr("CustomerId")),
.Name = sdr("Name").ToString(),
.Country = sdr("Country").ToString()
})
End While
con.Close()
End Using
Return customers
End Function
<WebMethod>
Public Function AddCustomer(ByVal Name As String, ByVal Country As String) As String
Dim status As String = ""
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "INSERT INTO Customers VALUES(@Name,@Country)"
Using con As SqlConnection = New SqlConnection(conString)
Dim cmd As SqlCommand = New SqlCommand(query)
cmd.Connection = con
cmd.Parameters.AddWithValue("@Name", Name)
cmd.Parameters.AddWithValue("@Country", Country)
con.Open()
status = If(cmd.ExecuteNonQuery() > 0, "true", "false")
con.Close()
End Using
Return status
End Function
Public Class Customer
Public Property Id As Integer
Public Property Name As String
Public Property Country As String
End Class
End Class
Screenshot
