You can display Google Maps inside GridView in following way.
Database
Table named as Locations.
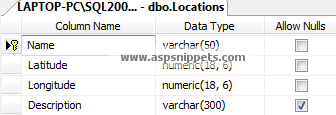
In the above table I have inserted Longitude and Latitude information of three different cities, along with their names and descriptions.
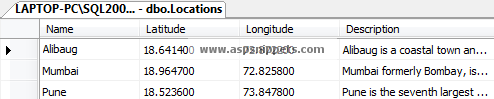
Download Database SQL
HTML
<asp:GridView ID="gvMaps" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" ItemStyle-CssClass="Name" />
<asp:BoundField DataField="Latitude" HeaderText="Latitude" ItemStyle-CssClass="Latitude" />
<asp:BoundField DataField="Longitude" HeaderText="Longitude" ItemStyle-CssClass="Longitude" />
<asp:TemplateField>
<ItemTemplate>
<div class="GoogleMap" style="height: 150px; width: 150px">
</div>
<span class="Description" style="display: none">
<%# Eval("Description") %></span>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Namespaces
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
Code
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
DataTable dt = this.GetData("SELECT * FROM Locations");
gvMaps.DataSource = dt;
gvMaps.DataBind();
}
}
private DataTable GetData(string query)
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
SqlCommand cmd = new SqlCommand(query);
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
sda.SelectCommand = cmd;
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
return dt;
}
}
}
}
Script
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?key=<API Key>"></script>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$("[id*=gvMaps] TR").each(function (index) {
if (index > 0) {
var marker = {
title: $(this).find(".Name").html(),
lat: $(this).find(".Latitude").html(),
lng: $(this).find(".Longitude").html(),
description: $(this).find(".Description").html()
};
var dvMap = $(this).find(".GoogleMap")[0];
LoadMap(marker, dvMap);
}
});
});
function LoadMap(marker, dvMap) {
var mapOptions = {
center: new google.maps.LatLng(marker.lat, marker.lng),
zoom: 8,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var infoWindow = new google.maps.InfoWindow();
var map = new google.maps.Map(dvMap, mapOptions);
var myLatlng = new google.maps.LatLng(marker.lat, marker.lng);
var m = new google.maps.Marker({
position: myLatlng,
map: map,
title: marker.title
});
(function (m, data) {
google.maps.event.addListener(marker, "click", function (e) {
infoWindow.setContent(marker.description);
infoWindow.open(map, marker);
});
})(m, marker);
};
</script>
Screenshot
