Hi dorsa,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
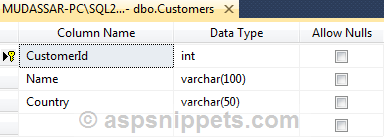
I have already inserted few records in the table.
You can download the database table SQL by clicking the download link below.
Download SQL file
SQL
CREATE PROCEDURE GetCustomersDetail
@Country VARCHAR(10)
AS
BEGIN
SELECT CustomerId,Name,Country,'false' AS IsChecked
FROM Customers
WHERE Country = @Country
END
HTML
<asp:Label Text="India" ID="lblCountry" runat="server" />
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:TemplateField HeaderText="Selectdata">
<ItemTemplate>
<asp:CheckBox ID="chkSelect" runat="server" Checked='<%# Convert.ToBoolean(Eval("IsChecked").ToString()) %>'
OnCheckedChanged="OnCheckedChanged" AutoPostBack="true" />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt;
if (ViewState["Data"] == null)
{
dt = GetData();
}
else
{
dt = ViewState["Data"] as DataTable;
}
GridView1.DataSource = dt;
GridView1.DataBind();
ViewState["Data"] = dt;
}
private DataTable GetData()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
SqlCommand cmd = new SqlCommand("GetCustomersDetail");
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlDataAdapter sda = new SqlDataAdapter())
{
cmd.Connection = con;
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.AddWithValue("@Country", lblCountry.Text.Trim());
sda.SelectCommand = cmd;
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
return dt;
}
}
}
}
protected void OnCheckedChanged(object sender, EventArgs e)
{
GridViewRow selectedRow = (sender as CheckBox).NamingContainer as GridViewRow;
string selectedName = selectedRow.Cells[1].Text;
int index = selectedRow.RowIndex;
if (ViewState["Data"] != null)
{
DataTable dt = ViewState["Data"] as DataTable;
dt.Rows[index]["IsChecked"] = (sender as CheckBox).Checked;
if ((sender as CheckBox).Checked)
{
int duplicateCount = dt.Select("Name='" + selectedName + "'").Length;
if (duplicateCount > 0)
{
for (int i = 0; i < dt.Rows.Count; i++)
{
if (i != index)
{
if (dt.Rows[i]["Name"].ToString() == selectedName)
{
dt.Rows[i]["IsChecked"] = false;
}
}
}
}
}
ViewState["Data"] = dt;
}
BindGridView();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
BindGridView()
End If
End Sub
Private Sub BindGridView()
Dim dt As DataTable
If ViewState("Data") Is Nothing Then
dt = GetData()
Else
dt = TryCast(ViewState("Data"), DataTable)
End If
GridView1.DataSource = dt
GridView1.DataBind()
ViewState("Data") = dt
End Sub
Private Function GetData() As DataTable
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim cmd As SqlCommand = New SqlCommand("GetCustomersDetail")
Using con As SqlConnection = New SqlConnection(conString)
Using sda As SqlDataAdapter = New SqlDataAdapter()
cmd.Connection = con
cmd.CommandType = CommandType.StoredProcedure
cmd.Parameters.AddWithValue("@Country", lblCountry.Text.Trim())
sda.SelectCommand = cmd
Using dt As DataTable = New DataTable()
sda.Fill(dt)
Return dt
End Using
End Using
End Using
End Function
Protected Sub OnCheckedChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim selectedRow As GridViewRow = TryCast((TryCast(sender, CheckBox)).NamingContainer, GridViewRow)
Dim selectedName As String = selectedRow.Cells(1).Text
Dim index As Integer = selectedRow.RowIndex
If ViewState("Data") IsNot Nothing Then
Dim dt As DataTable = TryCast(ViewState("Data"), DataTable)
dt.Rows(index)("IsChecked") = (TryCast(sender, CheckBox)).Checked
If (TryCast(sender, CheckBox)).Checked Then
Dim duplicateCount As Integer = dt.[Select]("Name='" & selectedName & "'").Length
If duplicateCount > 0 Then
For i As Integer = 0 To dt.Rows.Count - 1
If i <> index Then
If dt.Rows(i)("Name").ToString() = selectedName Then
dt.Rows(i)("IsChecked") = False
End If
End If
Next
End If
End If
ViewState("Data") = dt
End If
BindGridView()
End Sub
Screenshot
