Hi Hjalmaar,
Please refer below sample.
Database
For this example I have used database named FruitsDB which has a table named Fruits with the schema as follows.
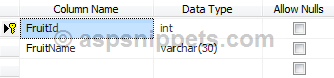
In the Fruits Table I have inserted few records as shown below.
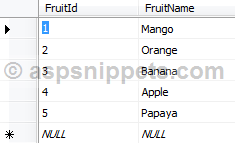
Model
FruitModel
public class FruitModel
{
[Key]
public int FruitId { get; set; }
public string FruitName { get; set; }
}
FruitListModel
public class FruitList
{
public List<SelectListItem> FruitsLists { get; set; }
}
Namespaces
using Bind_DropDownList_Core_Model.Model;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using System.Collections.Generic;
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
FruitList fruits = new FruitList()
{
FruitsLists = PopulateFruits()
};
return View(fruits);
}
private List<SelectListItem> PopulateFruits()
{
List<SelectListItem> fruits = new List<SelectListItem>();
foreach (FruitModel fruit in this.Context.Fruits.ToList())
{
fruits.Add(new SelectListItem { Text = fruit.FruitName, Value = fruit.FruitId.ToString() });
}
return fruits;
}
}
View
<select id="ddlFruits" asp-items="@Model.FruitsLists">
<option value="0">Please select</option>
</select>
Screenshot
