Hi Snooker,
Refer below sample.
Database
I have made use of the following table Customers with the schema as follows.
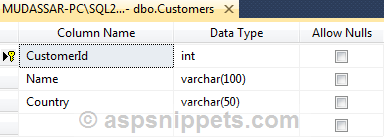
I have already inserted few records in the table.
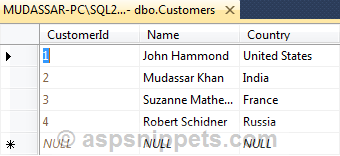
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:DetailsView ID="DetailsView1" runat="server" AutoGenerateRows="false" AllowPaging="true"
OnDataBound="OnDataBound" OnPageIndexChanging="OnPageIndexChanging">
<Fields>
<asp:BoundField DataField="CustomerId" HeaderText="Customer Id" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<asp:HiddenField ID="hfCountry" runat="server" Value='<%# Eval("Country") %>' />
<asp:DropDownList runat="server" ID="ddlCountries">
<asp:ListItem Text="Please Select" Value="0"></asp:ListItem>
<asp:ListItem Text="United States" Value="1"></asp:ListItem>
<asp:ListItem Text="India" Value="2"></asp:ListItem>
<asp:ListItem Text="France" Value="3"></asp:ListItem>
<asp:ListItem Text="Russia" Value="4"></asp:ListItem>
</asp:DropDownList>
</ItemTemplate>
</asp:TemplateField>
</Fields>
</asp:DetailsView>
Namespaces
C#
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Configuration
Imports System.Data
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid();
}
}
protected void OnDataBound(object sender, EventArgs e)
{
if (((DetailsView)sender).CurrentMode == DetailsViewMode.ReadOnly)
{
DataRowView row = (DataRowView)((DetailsView)sender).DataItem;
string country = ((HiddenField)((DetailsView)sender).FindControl("hfCountry")).Value;
DropDownList ddlCountries = (DropDownList)((DetailsView)sender).FindControl("ddlCountries");
if (ddlCountries.Items.FindByText(country) != null)
{
ddlCountries.Items.FindByText(country).Selected = true;
}
}
}
protected void OnPageIndexChanging(object sender, DetailsViewPageEventArgs e)
{
DetailsView1.PageIndex = e.NewPageIndex;
this.BindGrid();
}
private void BindGrid()
{
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con))
{
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
DetailsView1.DataSource = dt;
DetailsView1.DataBind();
}
}
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid()
End If
End Sub
Protected Sub OnDataBound(ByVal sender As Object, ByVal e As EventArgs)
If (CType(sender, DetailsView)).CurrentMode = DetailsViewMode.[ReadOnly] Then
Dim row As DataRowView = CType((CType(sender, DetailsView)).DataItem, DataRowView)
Dim country As String = (CType((CType(sender, DetailsView)).FindControl("hfCountry"), HiddenField)).Value
Dim ddlCountries As DropDownList = CType((CType(sender, DetailsView)).FindControl("ddlCountries"), DropDownList)
If ddlCountries.Items.FindByText(country) IsNot Nothing Then
ddlCountries.Items.FindByText(country).Selected = True
End If
End If
End Sub
Protected Sub OnPageIndexChanging(ByVal sender As Object, ByVal e As DetailsViewPageEventArgs)
DetailsView1.PageIndex = e.NewPageIndex
Me.BindGrid()
End Sub
Private Sub BindGrid()
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT CustomerId, Name, Country FROM Customers", con)
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
DetailsView1.DataSource = dt
DetailsView1.DataBind()
End Using
End Using
End Using
End Using
End Sub
Screenshot