Hi akhter,
Inorder to populate DropDownList with selected value you need to make use of Strongly Type model.
Check the below example.
Database
I have made use of the following table Customers with the schema as follows.
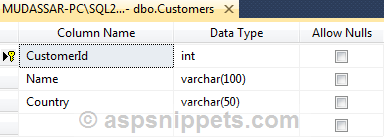
I have already inserted few records in the table.
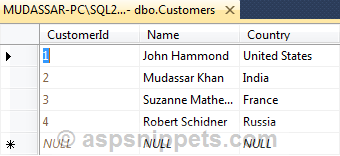
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
[Required]
public int Id { get; set; }
[Required]
public string Name { get; set; }
[Required]
public int CountryId { get; set; }
public SelectList Countries { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View(new AjaxSamplesEntities().Customers.ToList());
}
public ActionResult Edit(int? id)
{
AjaxSamplesEntities entities = new AjaxSamplesEntities();
Customer customer = entities.Customers.Find(id);
CustomerModel customerVM = new CustomerModel
{
Id = customer.CustomerId,
Name = customer.Name,
CountryId = customer.CustomerId,
Countries = new SelectList(entities.Customers.ToList(), "CustomerID", "Country", customer.CustomerId)
};
return View(customerVM);
}
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit(CustomerModel customerModel)
{
if (ModelState.IsValid)
{
// Update code goes here.
return RedirectToAction("Index");
}
else
{
AjaxSamplesEntities entities = new AjaxSamplesEntities();
customerModel.Countries = new SelectList(entities.Customers.ToList(), "CustomerID", "Country");
return View(customerModel);
}
}
}
View
Index
@model IEnumerable<Empty_DropDownList.Customer>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
</head>
<body class="container">
<table class="table table-responsive">
<tr>
<th>Name</th>
<th>Country</th>
<th>Action</th>
</tr>
@foreach (var customer in Model)
{
<tr>
<td>@customer.Name</td>
<td>@customer.Country</td>
<td>@Html.ActionLink("Edit", "Edit", new { id = customer.CustomerId })</td>
</tr>
}
</table>
</body>
</html>
Edit
@model Empty_DropDownList.Models.CustomerModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Edit</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
</head>
<body class="container">
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>Customer</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.Id)
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.CountryId, "Select Country", htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownList("CountryId", new SelectList(Model.Countries, "Value", "Text", Model.Id), "Select Country",
htmlAttributes: new { @class = "form-control", @id = "ddlCountries" })
@Html.ValidationMessageFor(model => model.CountryId, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
</body>
</html>
Screenshot
