Hi sunnyk21,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
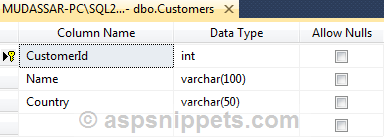
I have already inserted few records in the table.
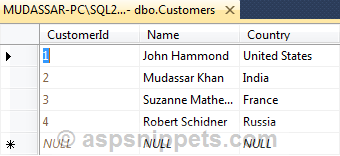
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Configuration;
using System.Data.SqlClient;
Model
public class UserModel
{
public int Id { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
TempData["Users"] = PopulateUsers();
return View();
}
[HttpPost]
public JsonResult GetUserDetails(string name)
{
UserModel model = new UserModel();
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT * FROM Customers WHERE Name = @Name";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Name", name);
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
if (sdr.Read())
{
model.Id = Convert.ToInt32(sdr["CustomerId"]);
model.Name = sdr["Name"].ToString();
model.Country = sdr["Country"].ToString();
}
}
con.Close();
}
}
return Json(model, JsonRequestBehavior.AllowGet);
}
[HttpPost]
public ActionResult Save(UserModel model)
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "UPDATE Customers SET Country = @Country WHERE Name = @Name";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Name", model.Name);
cmd.Parameters.AddWithValue("@Country", model.Country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
TempData["Users"] = PopulateUsers();
return View("Index");
}
private static List<SelectListItem> PopulateUsers()
{
List<SelectListItem> users = new List<SelectListItem>();
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "SELECT Name, CustomerId FROM Customers";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
con.Open();
using (SqlDataReader sdr = cmd.ExecuteReader())
{
while (sdr.Read())
{
users.Add(new SelectListItem
{
Text = sdr["Name"].ToString(),
Value = sdr["CustomerId"].ToString()
});
}
}
con.Close();
}
}
return users;
}
}
View
<%@ Page Language="C#" Inherits="System.Web.Mvc.ViewPage<_Edit_DropDown_Selected_Record.Models.UserModel>" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
function UserChanged() {
var userName = $('#ddlUsers option:selected').html();
$.ajax({
type: "POST",
url: "/Home/GetUserDetails",
data: "name=" + userName,
success: function (data) {
$('#Id').val(data.Id);
$('#Name').val(data.Name);
$('#Country').val(data.Country);
}
});
}
</script>
</head>
<body>
<% using (Html.BeginForm("Save", "Home", FormMethod.Post))
{%>
<table>
<tr>
<td>User</td>
<td><%: Html.DropDownList("ddlUsers", TempData["Users"] as List<SelectListItem>, "Select User", new { onchange = "UserChanged()" })%></td>
</tr>
<tr>
<td><%: Html.LabelFor(model => model.Id) %></td>
<td><%: Html.TextBoxFor(model => model.Id, new { @readonly = "readonly" })%>
<%: Html.HiddenFor(model => model.Name)%></td>
</tr>
<tr>
<td><%: Html.LabelFor(model => model.Country) %></td>
<td><%: Html.TextBoxFor(model => model.Country) %></td>
</tr>
</table>
<p><input type="submit" value="Save" /></p>
<% } %>
</body>
</html>
Screenshot
