Hi rani,
Use Aspose.Pdf library.
Check this example. Now please take its reference and correct your code.
Install the Aspose.Pdf from nuget.
Install-Package Aspose.PDF -Version 21.12.0
Database
I have made use of the following table Customers with the schema as follows.
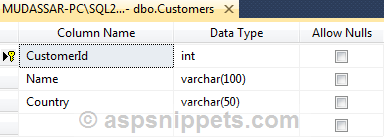
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
using System.Data;
using System.Data.SqlClient;
using System.IO;
using System.Text;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Aspose.Pdf.Text;
Controller
public class HomeController : Controller
{
private IHostingEnvironment Environment;
public HomeController(IHostingEnvironment _environment)
{
Environment = _environment;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult Index(IFormFile postedFile)
{
string path = System.IO.Path.Combine(this.Environment.WebRootPath, "Uploads");
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
string fileName = System.IO.Path.GetFileName(postedFile.FileName);
using (FileStream stream = new FileStream(System.IO.Path.Combine(path, fileName), FileMode.Create))
{
postedFile.CopyTo(stream);
}
DataTable dt = new DataTable();
Aspose.Pdf.Document pdfDocument = new Aspose.Pdf.Document(System.IO.Path.Combine(path, fileName));
foreach (var page in pdfDocument.Pages)
{
TableAbsorber absorber = new TableAbsorber();
absorber.Visit(page);
foreach (AbsorbedTable table in absorber.TableList)
{
int i = 0;
DataRow dr = null;
foreach (AbsorbedRow row in table.RowList)
{
int j = 0;
foreach (AbsorbedCell cell in row.CellList)
{
StringBuilder sb = new StringBuilder();
foreach (TextFragment fragment in cell.TextFragments)
{
foreach (TextSegment seg in fragment.Segments)
{
sb.Append(seg.Text);
}
}
if (i == 0)
{
dt.Columns.Add(sb.ToString().Trim());
j++;
if (row.CellList.Count == j)
{
dr = dt.NewRow();
j = 0;
i++;
}
}
else
{
dr[j] = sb.ToString().Trim();
j++;
if (row.CellList.Count == j)
{
dt.Rows.Add(dr.ItemArray);
j = 0;
i++;
}
}
}
}
}
}
string consString = @"Server=.;DataBase=AjaxSamples;UID=sa;PWD=pass@123";
using (SqlConnection con = new SqlConnection(consString))
{
using (SqlBulkCopy sqlBulkCopy = new SqlBulkCopy(con))
{
sqlBulkCopy.DestinationTableName = "dbo.Customers";
sqlBulkCopy.ColumnMappings.Add("Id", "CustomerId");
sqlBulkCopy.ColumnMappings.Add("Name", "Name");
sqlBulkCopy.ColumnMappings.Add("Country", "Country");
con.Open();
sqlBulkCopy.WriteToServer(dt);
con.Close();
}
}
return View();
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form method="post" enctype="multipart/form-data" asp-controller="Home" asp-action="Index">
<input type="file" name="postedFile" />
<input type="submit" value="Upload" />
</form>
</body>
</html>
For more details refer below link.
https://docs.aspose.com/pdf/net/extract-data-from-table-in-pdf/