Hi jmontano,
Using the article i have created the example.
Check this example. Now please take its reference and correct your code.
Database
For this example I have used table named Fruits whose schema is defined as follows.
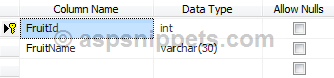
The Fruits table has the following records.
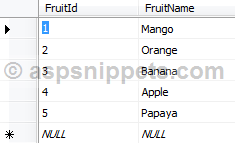
You can download the database table SQL by clicking the download link below.
Download SQL file
For reading connectrion string refer below article.
Model
using System.ComponentModel.DataAnnotations.Schema;
public class Fruit
{
public int FruitId { get; set; }
public string FruitName { get; set; }
[NotMapped]
public bool Selected { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View((from fruit in this.Context.Fruits
select new Fruit
{
FruitId = Convert.ToInt32(fruit.FruitId),
FruitName = fruit.FruitName.ToString()
}).ToList());
}
[HttpPost]
public IActionResult Index(List<Fruit> fruits)
{
ViewBag.Message = "";
foreach (Fruit fruit in fruits)
{
if (fruit.Selected)
{
ViewBag.Message += "Id : " + fruit.FruitId + " Name : " + fruit.FruitName + "\\n";
}
}
return View(fruits);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using CheckBoxList_Core_MVC.Models
@model List<Fruit>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<form asp-controller="Home" asp-action="Index" method="post">
@for (int i = 0; i < Model.Count; i++)
{
<div>
<input type="checkbox" asp-for="@Model[i].Selected" />
<label asp-for="@Model[i].FruitId">@Model[i].FruitName</label>
<input type="hidden" asp-for="@Model[i].FruitId" />
<input type="hidden" asp-for="@Model[i].FruitName" />
</div>
}
<br />
<input type="submit" value="Submit" />
</form>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
