Hi Jonsey ,
Refer below code.
Database
I have made use of the following table Customers with the schema as follows.
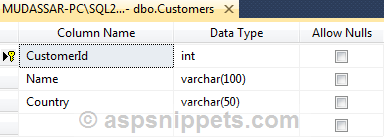
I have already inserted few records in the table.
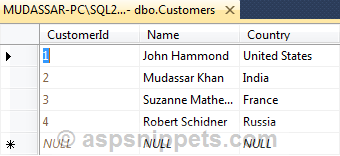
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
this.BindGrid();
}
}
private void BindGrid()
{
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
string query = "SELECT CustomerId,Name,Country FROM Customers";
using (SqlConnection con = new SqlConnection(constr))
{
using (SqlDataAdapter sda = new SqlDataAdapter(query, con))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
foreach (DataRow rs in dt.Rows)
{
if (rs["Name"] == DBNull.Value)
{
Response.Write("Value is Null" + "<br/>");
}
else if (string.IsNullOrEmpty(rs["Name"].ToString()))
{
Response.Write("Value is Empty" + "<br/>");
}
else
{
Response.Write("Value is not Null" + "<br/>");
}
}
}
}
}
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Me.BindGrid()
End If
End Sub
Private Sub BindGrid()
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Dim query As String = "SELECT CustomerId,Name,Country FROM Customers"
Using con As SqlConnection = New SqlConnection(constr)
Using sda As SqlDataAdapter = New SqlDataAdapter(query, con)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
For Each rs As DataRow In dt.Rows
If IsDBNull(rs("Name")) Then ' Null check
Response.Write("Value is Null" & "<br/>")
ElseIf String.IsNullOrEmpty(rs("Name").ToString()) Then ' Empty check
Response.Write("Value is Empty" & "<br/>")
Else
Response.Write("Value is not Null" & "<br/>")
End If
Next
End Using
End Using
End Using
End Sub