Hii RivickJoe,
Insert DropDownList selected value into database.
Please refer below sample.
Database Table
I have made use of the following table Customers with the schema as follows.
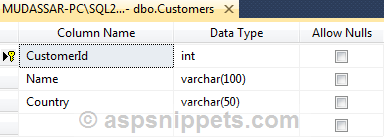
I have already inserted few records in the table.
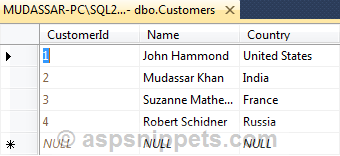
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<form id="form1" runat="server">
<div>
<asp:DropDownList ID="ddlCountry" runat="server">
<asp:ListItem Text="--Select--" Value="--Select--"></asp:ListItem>
<asp:ListItem Text="United States" Value="1"></asp:ListItem>
<asp:ListItem Text="India" Value="2"></asp:ListItem>
<asp:ListItem Text="France" Value="3"></asp:ListItem>
<asp:ListItem Text="Russia" Value="4"></asp:ListItem>
</asp:DropDownList>
<br />
<br />
<asp:Button ID="myBtn" runat="server" Text="Insert Data" OnClick="OnInsert" />
</div>
</form>
Namespaces
C#
using System.Data;
using System.Configuration;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
//OnClick function Insert Value in Database
protected void OnInsert(object sender, EventArgs e)
{
string selectedValue = ddlCountry.DataValueField;
string constring = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constring))
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO Customers (Country) VALUES (@Country)", con))
{
cmd.Parameters.AddWithValue("@Country", ddlCountry.SelectedValue);
con.Open();
int rowsAffected = cmd.ExecuteNonQuery();
if (rowsAffected > 0)
{
Response.Write(ddlCountry.SelectedValue);
}
else
{
Response.Write("Error Inserting Data");
}
}
}
}
VB.Net
'OnClick function Insert Value in Database
Protected Sub OnInsert(ByVal sender As Object, ByVal e As EventArgs)
Dim selectedValue As String = ddlCountry.DataValueField
Dim constring As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(constring)
Using cmd As SqlCommand = New SqlCommand("INSERT INTO Customers (Country) VALUES (@Country)", con)
cmd.Parameters.AddWithValue("@Country", ddlCountry.SelectedValue)
con.Open()
Dim rowsAffected As Integer = cmd.ExecuteNonQuery()
If rowsAffected > 0 Then
Response.Write(ddlCountry.SelectedValue)
Else
Response.Write("Error Inserting Data")
End If
End Using
End Using
End Sub
Screenshots
Inserting DropDownList value to Database

Inserted Data
