Hi rani,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
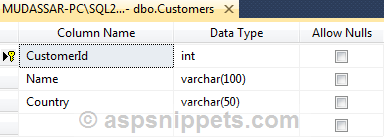
I have already inserted few records in the table.
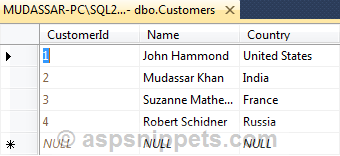
You can download the database table SQL by clicking the download link below.
Download SQL file
Controller
public class HomeController : Controller
{
// GET: /Home/
public ActionResult Index()
{
return View();
}
public JsonResult GetCustomers()
{
CustomerEntities entities = new CustomerEntities();
List<Customer> customers = entities.Customers.ToList();
return Json(customers, JsonRequestBehavior.AllowGet);
}
public JsonResult UpdateCustomer(Customer customer)
{
CustomerEntities entities = new CustomerEntities();
Customer customerForUpdate = entities.Customers.Where(x => x.CustomerId == customer.CustomerId).FirstOrDefault();
customerForUpdate.Name = customer.Name;
customerForUpdate.Country = customer.Country;
entities.SaveChanges();
return Json(customer, JsonRequestBehavior.AllowGet);
}
}
View
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Index</title>
<link rel="stylesheet" href="https://kendo.cdn.telerik.com/2020.1.114/styles/kendo.default-v2.min.css" />
<script type="text/javascript" src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<script type="text/javascript" src="https://kendo.cdn.telerik.com/2020.1.114/js/angular.min.js"></script>
<script type="text/javascript" src="https://kendo.cdn.telerik.com/2020.1.114/js/kendo.all.min.js"></script>
<style type="text/css">
body
{
font-family: Arial;
font-size: 10pt;
}
</style>
<script type="text/javascript">
var app = angular.module("MyApp", ["kendo.directives"]);
app.controller("MyController", function ($scope, $window, $http) {
$scope.mainGridOptions = {
dataSource: {
type: "json",
transport: {
read: { url: "Home/GetCustomers" },
update: { url: "Home/UpdateCustomer" }
},
schema: {
model: {
id: "CustomerId",
fields: {
CustomerId: { editable: false, nullable: true, type: "number" },
Name: { editable: true, nullable: true, type: "string" },
Country: { editable: true, nullable: true, type: "string" }
}
}
},
pageSize: 2,
serverPaging: false,
serverSorting: false
},
editable: "inline",
sortable: true,
pageable: true,
resizeable: true,
columns: [{ field: "CustomerId", title: "Id", width: "50px" },
{ field: "Name", title: "Name" },
{ field: "Country", title: "Country" },
{
title: 'Action',
command:
[
{ name: "edit", text: "Edit", iconClass: "k-icon k-i-hyperlink-open" }
]
}
]
};
})
</script>
</head>
<body ng-app="MyApp" ng-controller="MyController">
<kendo-grid k-options="mainGridOptions"></kendo-grid>
</body>
</html>
Screenshot
