Hi Akram.19,
The Binary data will be fetched from database and then will be converted into an Image object using ImageConverter class ConvertFrom method and will be displayed in PictureBox control.
Database
For this example i am making use of a table named tblFiles whose schema is defined as follows.
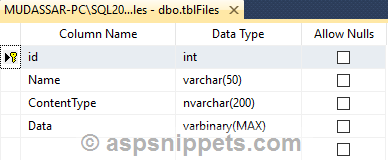
I have inserted few records in the table.
For more details of insert record in database refer below article.
you can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.Drawing;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Imports System.Drawing
Code
C#
private void View(object sender, EventArgs e)
{
if (pictureBox2.Image != null)
{
pictureBox2.Image.Dispose();
}
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("SELECT Data FROM tblFiles WHERE Id = 1", con))
{
cmd.CommandType = CommandType.Text;
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
byte[] bytes = (byte[])dt.Rows[0]["Data"];
ImageConverter converter = new ImageConverter();
Image img = (Image)converter.ConvertFrom(bytes);
pictureBox2.Image = img;
pictureBox2.SizeMode = PictureBoxSizeMode.Zoom;
pictureBox2.Refresh();
}
}
}
}
}
VB.Net
Private Sub btnView_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnView.Click
If pictureBox2.Image IsNot Nothing Then
pictureBox2.Image.Dispose()
End If
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("SELECT Data FROM tblFiles WHERE Id = 1", con)
cmd.CommandType = CommandType.Text
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
Dim bytes As Byte() = CType(dt.Rows(0)(0), Byte())
Dim converter As ImageConverter = New ImageConverter()
Dim img As Image = CType(converter.ConvertFrom(bytes), Image)
pictureBox2.Image = img
pictureBox2.SizeMode = PictureBoxSizeMode.Zoom
pictureBox2.Refresh()
End Using
End Using
End Using
End Using
End Sub
Screenshot
