Hi tsakumar81,
What issue you are facing while using Table Valued parameter in edmx file?
- Table Valued Parameters are only supported in raw/inline SQL in Entity Framework because we just pass any SqlParameter through to the underlying ADO.NET provider.
Here i have create a sample using the Table Valued parameter in Stored Procedure.
For configuring Stored Procedure in Entity Framework refer following article.
Call and execute a Stored Procedure in Entity Framework in ASP.Net using C# and VB.Net
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
Table Valued function
CREATE TYPE CustomerType AS TABLE
(
CustomerID NCHAR(5),
CompanyName NVARCHAR(40),
ContactName NVARCHAR(30),
City NVARCHAR(15),
Country NVARCHAR(15)
)
Stored Procedure
CREATE PROCEDURE Customers_InsertCustomer
@Customer CustomerType READONLY
AS
BEGIN
INSERT INTO Customers
(CustomerID
,CompanyName
,ContactName
,City
,Country)
SELECT CustomerID
,CompanyName
,ContactName
,City
,Country
FROM @Customer
END
Namespaces
using System.Data;
using System.Data.SqlClient;
Code
DataTable dtCustomers = new DataTable();
dtCustomers.Columns.Add("CustomerID", typeof(string));
dtCustomers.Columns.Add("CompanyName", typeof(string));
dtCustomers.Columns.Add("ContactName", typeof(string));
dtCustomers.Columns.Add("City", typeof(string));
dtCustomers.Columns.Add("Country", typeof(string));
dtCustomers.Rows.Add("DHARM", "ASPSnippets", "Dharmendra Das", "Mumbai", "India");
SqlParameter sqlParameter = new SqlParameter("@Customer", SqlDbType.Structured);
sqlParameter.Value = dtCustomers;
sqlParameter.TypeName = "dbo.CustomerType";
NorthwindEntities entities = new NorthwindEntities();
entities.ExecuteStoreCommand("exec dbo.Customers_InsertCustomer @Customer", sqlParameter);
If you do not want to use Table Valued parameter pass the Serialized Json string as parameter.
Here is an example.
DECLARE @json NVARCHAR(MAX) = N'[{ "CustomerID":"DHARM", "CompanyName":"ASPSnippets", "ContactName":"Dharmendra Das", "City":"Mumbai", "Country":"India"}]';
SELECT CustomerID
,CompanyName
,ContactName
,City
,Country
FROM OPENJSON(@json)
WITH (CustomerID NCHAR(5),
CompanyName NVARCHAR(40),
ContactName NVARCHAR(30),
City NVARCHAR(15),
Country NVARCHAR(15))
Output

For more details refer OPENJSON.
Screenshot
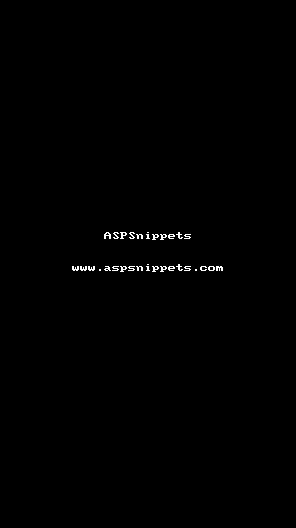
Downloads
Download Sample