Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used of Northwind database that you can download using the link given below.
Download Northwind Database
Model
public class Customer
{
public string CustomerID { get; set; }
public string ContactName { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View(this.Context.Customers.Take(10).ToList());
}
[HttpPost]
public IActionResult Export()
{
List<object> customers = (from customer in this.Context.Customers.Take(10).ToList()
select new[] {
customer.CustomerID,
customer.ContactName,
customer.City,
customer.Country
}).ToList<object>();
customers.Insert(0, new string[4] { "Customer ID", "Customer Name", "City", "Country" });
System.Text.StringBuilder sb = new System.Text.StringBuilder();
for (int i = 0; i < customers.Count; i++)
{
string[] customer = (string[])customers[i];
for (int j = 0; j < customer.Length; j++)
{
sb.Append(customer[j] + ',');
}
sb.Append("\r\n");
}
return File(System.Text.Encoding.UTF8.GetBytes(sb.ToString()), "text/csv", "Grid.csv");
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@using Export_Table_CSV_Core_MVC.Models
@model IEnumerable<Customer>
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<h4>Customers</h4>
<hr />
<div id="Grid">
<table>
<tr>
<th>Customer Id</th>
<th>Customer Name</th>
<th>City</th>
<th>Country</th>
</tr>
@foreach (Customer customer in Model)
{
<tr>
<td>@customer.CustomerID</td>
<td>@customer.ContactName</td>
<td>@customer.City</td>
<td>@customer.Country</td>
</tr>
}
</table>
</div>
<br />
<br />
<form asp-action="Export" asp-controller="Home" method="post">
<input type="submit" value="Export" />
</form>
</body>
</html>
Screenshots
HTML Table
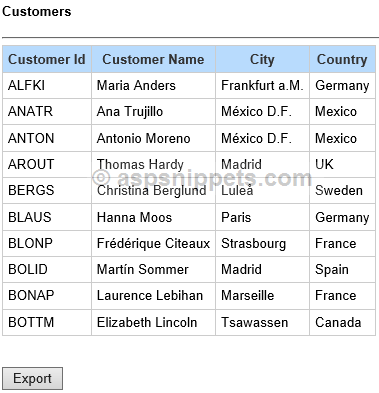
Exported CSV File
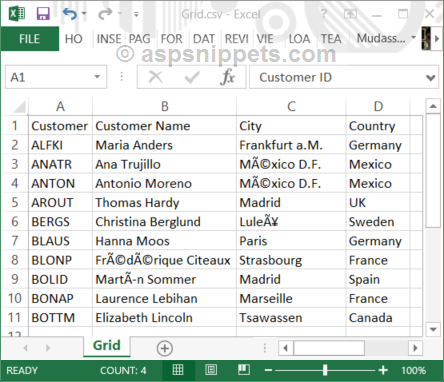