Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example I have used table named Fruits whose schema is defined as follows.
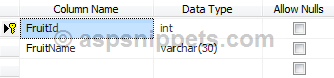
The Fruits table has the following records.
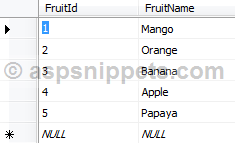
You can download the database table SQL by clicking the download link below.
Download SQL file
For entity framework configuration refer below article.
Model
Fruit
public class Fruit
{
public int FruitId { get; set; }
public string FruitName { get; set; }
}
Namespaces
using System.Collections.Generic;
using System.Linq;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.AspNetCore.Mvc.Rendering;
Razor PageModel code
public class IndexModel : PageModel
{
public string Message { get; set; }
public List<SelectListItem> Fruits { get; set; }
private DBCtx Context { get; }
public IndexModel(DBCtx _context)
{
this.Context = _context;
}
public void OnGet()
{
var fruits = (from fruit in this.Context.Fruits
select new SelectListItem
{
Text = fruit.FruitName,
Value = fruit.FruitId.ToString()
}).ToList();
this.Fruits = fruits;
}
public void OnPostSubmit()
{
var fruits = (from fruit in this.Context.Fruits
select new SelectListItem
{
Text = fruit.FruitName,
Value = fruit.FruitId.ToString()
}).ToList();
this.Fruits = fruits;
string[] fruitIds = Request.Form["lstFruits"].ToString().Split(",");
foreach (string id in fruitIds)
{
if (!string.IsNullOrEmpty(id))
{
string name = this.Fruits.Where(x => x.Value == id).FirstOrDefault().Text;
this.Message += "Id: " + id + " Fruit Name: " + name + "\\n";
}
}
}
}
Razor Page HTML
@page
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model Multiselect_DropDown_Core_Razor.Pages.IndexModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/css/bootstrap.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.0.3/js/bootstrap.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.rawgit.com/davidstutz/bootstrap-multiselect/master/dist/css/bootstrap-multiselect.css" />
<script type="text/javascript" src="https://cdn.rawgit.com/davidstutz/bootstrap-multiselect/master/dist/js/bootstrap-multiselect.js"></script>
<script type="text/javascript">
$(function () {
$('#lstFruits').multiselect({
includeSelectAllOption: true
});
});
</script>
</head>
<body>
<form method="post">
<div class="container">
Fruits:
<select id="lstFruits" name="lstFruits" asp-items="@Model.Fruits" multiple class="form-control"></select>
<input type="submit" value="Submit" asp-page-handler="Submit" class="btn btn-primary" />
</div>
</form>
@if (@Model.Message != null)
{
<script type="text/javascript">
window.onload = function () {
alert("@Model.Message");
};
</script>
}
</body>
</html>
Screenshot
