Hi trisetia302,
You need to change the default Camel Case JSON Output in ASP.Net Core.
For more details refer below article.
ASP.Net Core: Changing the default Camel Case JSON Output
Refer below example.
Database
I have made use of the following table Customers with the schema as follows.
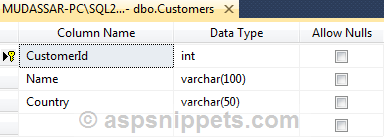
I have already inserted few records in the table.
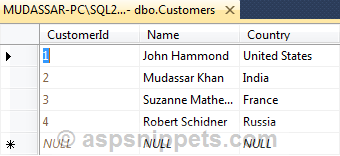
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View();
}
[HttpGet]
public async Task<JsonResult> GetCustomers(string term)
{
List<Customer> data = await this.Context.Customers
.Where(A => A.Name.ToUpper().Contains(term))
.ToListAsync();
return new JsonResult(data);
}
}
View
@addTagHelper*, Microsoft.AspNetCore.Mvc.TagHelpers
@model Select_DropDown_jQuery_MVC_Core.Models.Customer
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" id="SelectRegionalKTP" style="width: 100%;"
class="form-control js-example-basic-single">
</select>
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/css/select2.min.css" rel="stylesheet" />
<script src="https://cdn.jsdelivr.net/npm/select2@4.0.13/dist/js/select2.min.js"></script>
<script>
$(document).ready(function () {
$('#SelectRegionalKTP').select2({
minimumInputLength: 1,
ajax: {
url: '/Home/GetCustomers',
dataType: 'json',
data: function (params) {
return { term: params.term }
},
processResults: function (data) {
return {
results: $.map(data, function (item) {
return {
id: item.CustomerId,
text: item.Name
};
})
};
}
}
});
});
</script>
</body>
</html>
Screenshot
