Hi just123,
Refer below code. Since you are using master page you need to find the master page with the contentplaceholde and then find the gridview.
MasterPage.master
<%@ Master Language="C#" AutoEventWireup="true" CodeFile="MasterPage.master.cs" Inherits="MasterPage" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<asp:ContentPlaceHolder ID="head" runat="server">
</asp:ContentPlaceHolder>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ContentPlaceHolder ID="ContentPlaceHolder1" runat="server">
</asp:ContentPlaceHolder>
</div>
</form>
</body>
</html>
VB.Net
DefaultVB.aspx
<%@ Page Title="" Language="VB" MasterPageFile="~/MasterPage.master" AutoEventWireup="false"
CodeFile="DefaultVB.aspx.vb" Inherits="DefaultVB" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="Server">
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="Server">
<style type="text/css">
body
{
font-family: Arial;
font-size: 10pt;
}
</style>
<asp:GridView ID="GridView1" HeaderStyle-BackColor="#3AC0F2" HeaderStyle-ForeColor="White"
RowStyle-BackColor="#A1DCF2" AlternatingRowStyle-BackColor="White" AlternatingRowStyle-ForeColor="#000"
runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" ItemStyle-Width="30" />
<asp:TemplateField>
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Eval("Name") %>' />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Country" HeaderText="Country" ItemStyle-Width="150" />
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton ID="lnkDetails" runat="server" Text="Send Details" PostBackUrl='<%# "~/DetailsVB.aspx?RowIndex=" & Container.DataItemIndex %>'></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</asp:Content>
DetailsVB.aspx
<%@ Page Title="" Language="VB" MasterPageFile="~/MasterPage.master" AutoEventWireup="false"
CodeFile="DetailsVB.aspx.vb" Inherits="DetailsVB" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="Server">
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="Server">
<style type="text/css">
body
{
font-family: Arial;
font-size: 10pt;
}
</style>
<table>
<tr>
<td>
<b>Id</b>
</td>
<td>
<asp:Label ID="lblId" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Name</b>
</td>
<td>
<asp:Label ID="lblName" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Country</b>
</td>
<td>
<asp:Label ID="lblCountry" runat="server"></asp:Label>
</td>
</tr>
</table>
</asp:Content>
Code
DefaultVB.aspx.vb
Partial Class DefaultVB
Inherits System.Web.UI.Page
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
Dim dt As New DataTable()
dt.Columns.AddRange(New DataColumn(2) {New DataColumn("Id"), New DataColumn("Name"), New DataColumn("Country")})
dt.Rows.Add(1, "John Hammond", "United States")
dt.Rows.Add(2, "Mudassar Khan", "India")
dt.Rows.Add(3, "Suzanne Mathews", "France")
dt.Rows.Add(4, "Robert Schidner", "Russia")
GridView1.DataSource = dt
GridView1.DataBind()
End If
End Sub
End Class
DetailsVB.aspx.vb
Partial Class DetailsVB
Inherits System.Web.UI.Page
Protected Sub Page_Load(sender As Object, e As EventArgs) Handles Me.Load
If Me.Page.PreviousPage IsNot Nothing Then
Dim rowIndex As Integer = Integer.Parse(Request.QueryString("RowIndex"))
Dim GridView1 As GridView = DirectCast(Me.Page.PreviousPage.Master.FindControl("ContentPlaceHolder1").FindControl("GridView1"), GridView)
Dim row As GridViewRow = GridView1.Rows(rowIndex)
lblId.Text = row.Cells(0).Text
lblName.Text = CType(row.FindControl("lblName"), Label).Text
lblCountry.Text = row.Cells(2).Text
End If
End Sub
End Class
C#
DefaultCS.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/MasterPage.master" AutoEventWireup="true"
CodeFile="DefaultCS.aspx.cs" Inherits="_Default" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="Server">
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="Server">
<style type="text/css">
body
{
font-family: Arial;
font-size: 10pt;
}
</style>
<asp:GridView ID="GridView1" HeaderStyle-BackColor="#3AC0F2" HeaderStyle-ForeColor="White"
RowStyle-BackColor="#A1DCF2" AlternatingRowStyle-BackColor="White" AlternatingRowStyle-ForeColor="#000"
runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Id" HeaderText="Id" ItemStyle-Width="30" />
<asp:TemplateField>
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Eval("Name") %>' />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Country" HeaderText="Country" ItemStyle-Width="150" />
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton ID="lnkDetails" runat="server" Text="Send Details" PostBackUrl='<%# "~/DetailsCS.aspx?RowIndex=" + Container.DataItemIndex %>'></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</asp:Content>
DetailsCS.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/MasterPage.master" AutoEventWireup="true"
CodeFile="DetailsCS.aspx.cs" Inherits="Details" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="Server">
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" runat="Server">
<style type="text/css">
body
{
font-family: Arial;
font-size: 10pt;
}
</style>
<table>
<tr>
<td>
<b>Id</b>
</td>
<td>
<asp:Label ID="lblId" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Name</b>
</td>
<td>
<asp:Label ID="lblName" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td>
<b>Country</b>
</td>
<td>
<asp:Label ID="lblCountry" runat="server"></asp:Label>
</td>
</tr>
</table>
</asp:Content>
Code
DefaultCS.aspx.cs
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
DataTable dt = new DataTable();
dt.Columns.AddRange(new DataColumn[3] { new DataColumn("Id"), new DataColumn("Name"), new DataColumn("Country") });
dt.Rows.Add(1, "John Hammond", "United States");
dt.Rows.Add(2, "Mudassar Khan", "India");
dt.Rows.Add(3, "Suzanne Mathews", "France");
dt.Rows.Add(4, "Robert Schidner", "Russia");
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
DetailsCS.aspx.cs
public partial class Details : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (this.Page.PreviousPage != null)
{
int rowIndex = int.Parse(Request.QueryString["RowIndex"]);
GridView GridView1 = (GridView)this.Page.PreviousPage.Master.FindControl("ContentPlaceHolder1").FindControl("GridView1");
GridViewRow row = GridView1.Rows[rowIndex];
lblId.Text = row.Cells[0].Text;
lblName.Text = (row.FindControl("lblName") as Label).Text;
lblCountry.Text = row.Cells[2].Text;
}
}
}
Output
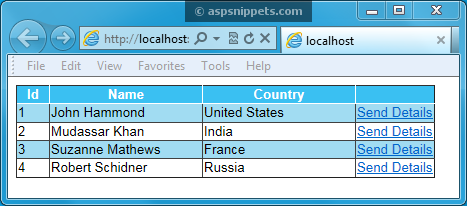
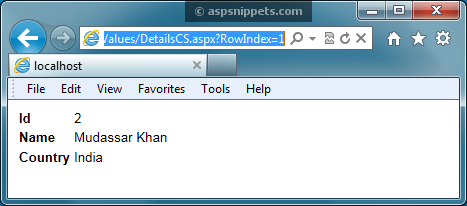