Dera Indradeo,
You didn't set the DataKeyNames. You need to set the GridView DataKeyNames property.
Kindly refer below Sample.
Database
I have made use of the following table Customers with the schema as follows.
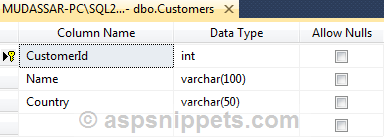
I have already inserted few records in the table.
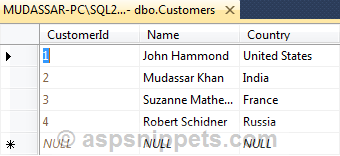
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" OnRowUpdating="GridView1_RowUpdating" DataKeyNames="CustomerId"
BackColor="White" Width="1050px" BorderColor="#CCCCCC" OnRowCancelingEdit="GridView1_RowCancelingEdit" OnRowEditing="GridView1_RowEditing"
BorderStyle="None" BorderWidth="1px" CellPadding="3" CssClass="auto-style16" Height="170px" OnRowDataBound="GridView1_RowDataBound">
<Columns>
<asp:TemplateField HeaderText="SL.No." ItemStyle-Width="10">
<ItemTemplate>
<asp:Label ID="lblRowNumber" Text='<%# Container.DataItemIndex + 1 %>' runat="server" />
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Customer Id">
<ItemTemplate>
<asp:Label ID="lblId" runat="server" Text='<%# Eval("CustomerId") %>' ></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<%--<asp:Label ID="Name" runat="server" Text='<%# Eval("Name") %>'></asp:Label>--%>
<asp:DropDownList runat="server" ID="ddlName">
</asp:DropDownList>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<%--<asp:Label ID="country" runat="server" Text='<%# Eval("Country") %>'></asp:Label>--%>
<asp:DropDownList runat="server" ID="ddlCountries">
</asp:DropDownList>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField>
<ItemTemplate>
<asp:Button ID="btnUpdate" runat="server" CommandName="Update" Text="Update"></asp:Button>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField>
<ItemTemplate>
<asp:Button ID="Button3" runat="server" CommandName="Cancel" Text="Cancel"></asp:Button>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="White" ForeColor="#000066" />
<HeaderStyle BackColor="#006699" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="White" ForeColor="#000066" HorizontalAlign="Left" />
<RowStyle ForeColor="#000066" />
<SelectedRowStyle BackColor="#669999" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#F1F1F1" />
<SortedAscendingHeaderStyle BackColor="#007DBB" />
<SortedDescendingCellStyle BackColor="#CAC9C9" />
<SortedDescendingHeaderStyle BackColor="#00547E" />
</asp:GridView>
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGrid();
}
}
// This method is used to bind gridview from database
private void BindGrid()
{
using (SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ConnectionString))
{
//SqlDataAdapter sda = new SqlDataAdapter("SELECT * FROM comp_box order by COMP_LODGE_DATE DESC ", con);
using (SqlDataAdapter sda = new SqlDataAdapter("SELECT CustomerId, Name, Country From Customers", con))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
}
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
SqlConnection con = new SqlConnection(ConfigurationManager.ConnectionStrings["constr"].ConnectionString);
if (e.Row.RowType == DataControlRowType.DataRow)
{
using (SqlCommand cmd = new SqlCommand("select Name from Customers", con))
{
con.Open();
DropDownList ddlName = (e.Row.FindControl("ddlName") as DropDownList);
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
con.Close();
ddlName.DataSource = dt;
ddlName.DataTextField = "Name";
ddlName.DataValueField = "Name";
ddlName.DataBind();
ddlName.Items.Insert(0, new ListItem("--Select Name--", "0"));
}
}
}
using (SqlCommand cmd = new SqlCommand("select Country from Customers", con))
{
con.Open();
DropDownList ddlCountries = (e.Row.FindControl("ddlCountries") as DropDownList);
using (SqlDataAdapter sda = new SqlDataAdapter(cmd))
{
using (DataTable dt = new DataTable())
{
sda.Fill(dt);
con.Close();
ddlCountries.DataSource = dt;
ddlCountries.DataTextField = "Country";
ddlCountries.DataValueField = "Country";
ddlCountries.DataBind();
ddlCountries.Items.Insert(0, new ListItem("--Select Country--", "0"));
}
}
}
}
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
string id = GridView1.DataKeys[e.RowIndex].Value.ToString();
string name = (GridView1.Rows[e.RowIndex].FindControl("ddlName") as DropDownList).SelectedItem.Value;
string country = (GridView1.Rows[e.RowIndex].FindControl("ddlCountries") as DropDownList).SelectedItem.Value;
string strConnString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(strConnString))
{
string query = "update Customers set Name = @Name,Country=@Country where CustomerID = @Id";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Id", id);
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
BindGrid();
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
BindGrid();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
BindGrid()
End If
End Sub
Private Sub BindGrid()
Using con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("constr").ConnectionString)
Using sda As SqlDataAdapter = New SqlDataAdapter("SELECT CustomerId, Name, Country From Customers", con)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
GridView1.DataSource = dt
GridView1.DataBind()
End Using
End Using
End Using
End Sub
Protected Sub GridView1_RowDataBound(ByVal sender As Object, ByVal e As GridViewRowEventArgs)
Dim con As SqlConnection = New SqlConnection(ConfigurationManager.ConnectionStrings("constr").ConnectionString)
If e.Row.RowType = DataControlRowType.DataRow Then
Using cmd As SqlCommand = New SqlCommand("select Name from Customers", con)
con.Open()
Dim ddlName As DropDownList = (TryCast(e.Row.FindControl("ddlName"), DropDownList))
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
con.Close()
ddlName.DataSource = dt
ddlName.DataTextField = "Name"
ddlName.DataValueField = "Name"
ddlName.DataBind()
ddlName.Items.Insert(0, New ListItem("--Select Name--", "0"))
End Using
End Using
End Using
Using cmd As SqlCommand = New SqlCommand("select Country from Customers", con)
con.Open()
Dim ddlCountries As DropDownList = (TryCast(e.Row.FindControl("ddlCountries"), DropDownList))
Using sda As SqlDataAdapter = New SqlDataAdapter(cmd)
Using dt As DataTable = New DataTable()
sda.Fill(dt)
con.Close()
ddlCountries.DataSource = dt
ddlCountries.DataTextField = "Country"
ddlCountries.DataValueField = "Country"
ddlCountries.DataBind()
ddlCountries.Items.Insert(0, New ListItem("--Select Country--", "0"))
End Using
End Using
End Using
End If
End Sub
Protected Sub GridView1_RowUpdating(ByVal sender As Object, ByVal e As GridViewUpdateEventArgs)
Dim id As String = GridView1.DataKeys(e.RowIndex).Value.ToString()
Dim name As String = (TryCast(GridView1.Rows(e.RowIndex).FindControl("ddlName"), DropDownList)).SelectedItem.Value
Dim country As String = (TryCast(GridView1.Rows(e.RowIndex).FindControl("ddlCountries"), DropDownList)).SelectedItem.Value
Dim strConnString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(strConnString)
Dim query As String = "update Customers set Name = @Name,Country=@Country where CustomerID = @Id"
Using cmd As SqlCommand = New SqlCommand(query)
cmd.Connection = con
cmd.Parameters.AddWithValue("@Id", id)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
End Sub
Protected Sub GridView1_RowCancelingEdit(ByVal sender As Object, ByVal e As GridViewCancelEditEventArgs)
GridView1.EditIndex = -1
BindGrid()
End Sub
Protected Sub GridView1_RowEditing(ByVal sender As Object, ByVal e As GridViewEditEventArgs)
GridView1.EditIndex = e.NewEditIndex
BindGrid()
End Sub
Screenshot

Database After Update
