Hi Jilsoft,
Please refer below sample.
Database
This sample makes use of a table named Files whose schema is defined as follows.
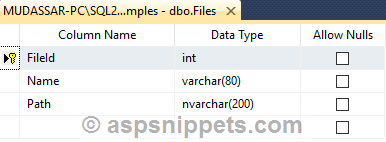
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<div>
<table border="0" cellpadding="0" cellspacing="0">
<tr>
<td>
<asp:FileUpload ID="FileUpload1" runat="server" /></td>
</tr>
<tr>
<td colspan="2">
<asp:Button ID="btnSave" Text="Save" runat="server" CssClass="btn btn-primary" /></td>
</tr>
</table>
</div>
<div>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<script type="text/javascript" src="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
<script type="text/javascript">
$(function () {
var reader = new FileReader();
var fileName;
var contentType;
$('[id*=FileUpload1]').change(function () {
if (typeof (FileReader) != "undefined") {
var regex = /^([a-zA-Z0-9\s_\\.\-:])+(.jpg|.jpeg|.gif|.png|.bmp)$/;
$($(this)[0].files).each(function () {
var file = $(this);
if (regex.test(file[0].name.toLowerCase())) {
fileName = file[0].name;
contentType = file[0].type;
reader.readAsDataURL(file[0]);
} else {
alert(file[0].name + " is not a valid image file.");
return false;
}
});
} else {
alert("This browser does not support HTML5 FileReader.");
}
});
$("[id*=btnSave]").click(function () {
var byteData = reader.result;
byteData = byteData.split(';')[1].replace("base64,", "");
var obj = {};
obj.Data = byteData;
obj.Name = fileName;
obj.ContentType = contentType;
$.ajax({
type: "POST",
url: "WebService.asmx/SaveImage",
data: '{data : ' + JSON.stringify(obj) + ' }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
alert(r.d);
},
error: function (r) {
alert(r.responseText);
},
failure: function (r) {
alert(r.responseText);
}
});
return false;
});
});
</script>
</div>
Code
WebService
C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Configuration;
using System.Data.SqlClient;
using System.IO;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class WebService : System.Web.Services.WebService
{
[WebMethod]
public string SaveImage(FileData data)
{
string filePath = Server.MapPath("~/Files/");
if (!Directory.Exists(filePath))
{
Directory.CreateDirectory(filePath);
}
string name = Path.GetFileName(data.Name);
byte[] bytes = Convert.FromBase64String(data.Data);
File.WriteAllBytes(filePath + name, bytes);
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection conn = new SqlConnection(constr))
{
string sql = "INSERT INTO tblFilePath VALUES(@Name, @Path)";
using (SqlCommand cmd = new SqlCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Path", "~/Files/" + name);
conn.Open();
cmd.ExecuteNonQuery();
conn.Close();
}
}
return "Data Saved Successfully";
}
public class FileData
{
public string Data { get; set; }
public string ContentType { get; set; }
public string Name { get; set; }
}
}
VB.Net
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web
Imports System.Web.Services
Imports System.Configuration
Imports System.Data.SqlClient
Imports System.IO
' To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
<System.Web.Script.Services.ScriptService()>
<WebService(Namespace:="http://tempuri.org/")>
<WebServiceBinding(ConformsTo:=WsiProfiles.BasicProfile1_1)>
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()>
Public Class WebService
Inherits System.Web.Services.WebService
<WebMethod>
Public Function SaveImage(ByVal data As FileData) As String
Dim filePath As String = Server.MapPath("~/Files/")
If Not Directory.Exists(filePath) Then
Directory.CreateDirectory(filePath)
End If
Dim name As String = Path.GetFileName(data.Name)
Dim bytes As Byte() = Convert.FromBase64String(data.Data)
File.WriteAllBytes(filePath & name, bytes)
Dim constr As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using conn As SqlConnection = New SqlConnection(constr)
Dim sql As String = "INSERT INTO tblFilePath VALUES(@Name, @Path)"
Using cmd As SqlCommand = New SqlCommand(sql, conn)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Path", "~/Files/" & name)
conn.Open()
cmd.ExecuteNonQuery()
conn.Close()
End Using
End Using
Return "Data Saved Successfully"
End Function
Public Class FileData
Public Property Data As String
Public Property ContentType As String
Public Property Name As String
End Class
End Class
Screenshot
