Hi ramco1917,
Your view is declared IEnumerable of entity as model.
You can't pass single object to View. Create another class with all the columns as properties and Generic list of entity model.
Then use the Class as model for the view.
Set the input textbox name attribute same as Model property name.
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
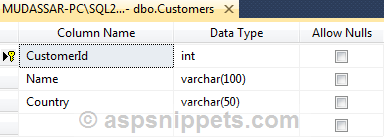
I have already inserted few records in the table.
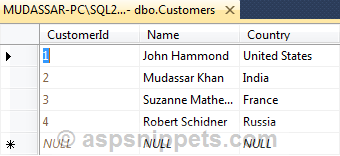
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class CustomerModel
{
public int CustomerID { get; set; }
public string Name { get; set; }
public string Country { get; set; }
public List<Customer> Customers { get; set; }
}
Controller
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
using (AjaxSamplesEntities entities = new AjaxSamplesEntities())
{
CustomerModel model = new CustomerModel();
model.Customers = entities.Customers.ToList();
return View(model);
}
}
[HttpPost]
public ActionResult Index(CustomerModel model)
{
using (AjaxSamplesEntities entities = new AjaxSamplesEntities())
{
if (model.CustomerID > 0)
{
Customer customerUpdate = entities.Customers.Where(x => x.CustomerId == model.CustomerID).FirstOrDefault();
customerUpdate.Name = model.Name;
customerUpdate.Country = model.Country;
entities.SaveChanges();
}
else
{
entities.Customers.Add(new Customer
{
Name = model.Name,
Country = model.Country
});
entities.SaveChanges();
}
model.Customers = entities.Customers.ToList();
return View(model);
}
}
public ActionResult DeleteCustomer(int id)
{
using (AjaxSamplesEntities entities = new AjaxSamplesEntities())
{
Customer customer = entities.Customers.Where(x => x.CustomerId == id).FirstOrDefault();
entities.Customers.Remove(customer);
entities.SaveChanges();
CustomerModel model = new CustomerModel();
model.Name = customer.Name;
model.Country = customer.Country;
model.Customers = entities.Customers.ToList();
return View("Index", model);
}
}
}
View
@model jQuery_DataTable_MVC.Models.CustomerModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
<script type="text/javascript" src="https://code.jquery.com/jquery-3.2.1.slim.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.20/js/jquery.dataTables.min.js"></script>
<link href="https://cdn.datatables.net/1.10.20/css/jquery.dataTables.css" rel="stylesheet" type="text/css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" />
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" />
<script type="text/javascript" src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<script type="text/javascript">
$(function () {
$("#tblCustomers").dataTable();
$('[id*=btnEdit]').on('click', function () {
var tds = $(this).closest('tr').find('td');
$('#hfId').val($(tds).eq(0).html());
$('#txtName').val($(tds).eq(1).html());
$('#txtCountry').val($(tds).eq(2).html());
$('#myModal').modal('show');
});
});
</script>
</head>
<body class="container">
<br />
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#myModal"> <i class="fa fa-plus"></i> Add New</button><br /><br />
<table class="table" id="tblCustomers">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
<th>Action</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model.Customers)
{
<tr>
<td>@Html.DisplayFor(modelItem => item.CustomerId)</td>
<td>@Html.DisplayFor(modelItem => item.Name)</td>
<td>@Html.DisplayFor(modelItem => item.Country)</td>
<td>
<a class='btn btn-primary btn-sm' id='btnEdit'><i class='fa fa-pencil'></i> Edit</a>
<a href="@Url.Action("DeleteCustomer","Home")/@item.CustomerId" onclick="return confirm('Do you want to delete this record?')"
class='btn btn-danger btn-sm' id='btnDelete' style='margin-left:5px'><i class='fa fa-trash'></i> Delete</a>
</td>
</tr>
}
</tbody>
</table>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
<div id="myModal" class="modal" tabindex="-1" role="dialog">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Customer Details Form</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="form-group">
<input type="hidden" id="hfId" name="CustomerID" />
<label>Name:</label>
<input type="text" id="txtName" name="Name" value="" class="form-control" />
</div>
<div class="form-group">
<label>Country:</label>
<input type="text" id="txtCountry" name="Country" value="" class="form-control" />
</div>
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-primary">Save changes</button>
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
}
</body>
</html>
Screenshot
