Hi rani,
Check this example. Now please take its reference and correct your code.
Controller
public class HomeController : Controller
{
// GET: Home
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult Index(string txtAddress, string txtLatitude, string txtLongitude)
{
ViewBag.Message = "Address: " + txtAddress;
ViewBag.Message += "\\nLatitude: " + txtLatitude;
ViewBag.Message += "\\nLongitude: " + txtLongitude;
return View();
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
@using (Html.BeginForm("Index", "Home", FormMethod.Post))
{
<table border="0" cellpadding="5" cellspacing="0">
<tr>
<td>Location</td>
<td><input type="text" id="txtLocation" name="txtLocation" /></td>
</tr>
<tr>
<td>Address</td>
<td><input type="text" id="txtAddress" name="txtAddress" /></td>
</tr>
<tr>
<td>Latitude</td>
<td><input type="text" id="txtLatitude" name="txtLatitude" /></td>
</tr>
<tr>
<td>Longitude</td>
<td><input type="text" id="txtLongitude" name="txtLongitude" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="Submit" /></td>
</tr>
</table>
}
<script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?sensor=false&libraries=places&key=<API Key>"></script>
<script type="text/javascript">
google.maps.event.addDomListener(window, 'load', function () {
var places = new google.maps.places.Autocomplete(document.getElementById('txtLocation'));
google.maps.event.addListener(places, 'place_changed', function () {
var place = places.getPlace();
document.getElementById('txtAddress').value = place.formatted_address;
document.getElementById('txtLatitude').value = place.geometry.location.lat();
document.getElementById('txtLongitude').value = place.geometry.location.lng();
});
});
</script>
@if (ViewBag.Message != null)
{
<script type="text/javascript">
window.onload = function(){
alert("@ViewBag.Message");
};
</script>
}
</body>
</html>
Screenshot
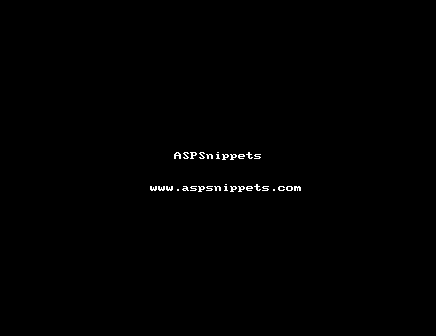