Hi rani,
Check this example. Now please take its reference and correct your code.
Database
For this example i have used two tables Countries, State with the following schema.
Countries Table
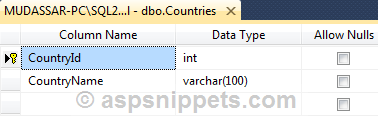
States Table
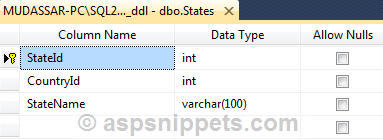
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.6.8/angular.min.js"></script>
<script type="text/javascript">
var app = angular.module('MyApp', []);
app.controller('MyController', function ($scope, $http, $location, $anchorScroll) {
$http.post('Default.aspx/GetData', { headers: { 'Content-Type': 'application/json'} })
.then(function (response) {
$scope.Countries = response.data.d;
});
$scope.ScrollTo = function (countryName) {
$location.hash(countryName);
$anchorScroll();
}
});
</script>
<div ng-app="MyApp" ng-controller="MyController">
<table>
<tr>
<td ng-repeat="country in Countries">
<button type="button" ng-click="ScrollTo(country.Name)">
{{country.Name}}</button>
</td>
</tr>
</table>
<hr />
<ul ng-repeat="country in Countries" id="{{country.Name}}">
<h3>
{{country.Name}}</h3>
<li ng-repeat="state in country.States">{{state.Name}} </li>
</ul>
</div>
Namespaces
C#
using System.Collections.Generic;
using System.Linq;
using System.Web.Services;
using CascadingModel;
VB.Net
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web.Services
Imports CascadingModel
Code
C#
[WebMethod]
public static List<Countries> GetData()
{
CascadingEntities entities = new CascadingEntities();
List<Countries> listCountries = new List<Countries>();
foreach (Country country in entities.Countries)
{
listCountries.Add(new Countries
{
Id = country.CountryId,
Name = country.CountryName,
States = GetStates(country.CountryId)
});
}
return listCountries;
}
public static List<States> GetStates(int countryId)
{
CascadingEntities entities = new CascadingEntities();
List<States> listStates = new List<States>();
List<State> states = entities.States.Where(x => x.CountryId == countryId).ToList();
foreach (State state in states)
{
listStates.Add(new States { Id = state.StateId, Name = state.StateName });
}
return listStates;
}
public class Countries
{
public int Id { get; set; }
public string Name { get; set; }
public List<States> States { get; set; }
}
public class States
{
public int Id { get; set; }
public string Name { get; set; }
}
VB.Net
<WebMethod()>
Public Shared Function GetData() As List(Of Countries)
Dim entities As CascadingEntities = New CascadingEntities()
Dim listCountries As List(Of Countries) = New List(Of Countries)()
For Each country As Country In entities.Countries
listCountries.Add(New Countries With {
.Id = country.CountryId,
.Name = country.CountryName,
.States = GetStates(country.CountryId)
})
Next
Return listCountries
End Function
Public Shared Function GetStates(ByVal countryId As Integer) As List(Of States)
Dim entities As CascadingEntities = New CascadingEntities()
Dim listStates As List(Of States) = New List(Of States)()
Dim states As List(Of State) = entities.States.Where(Function(x) x.CountryId = countryId).ToList()
For Each state As State In states
listStates.Add(New States With {
.Id = state.StateId,
.Name = state.StateName
})
Next
Return listStates
End Function
Public Class Countries
Public Property Id As Integer
Public Property Name As String
Public Property States As List(Of States)
End Class
Public Class States
Public Property Id As Integer
Public Property Name As String
End Class
Screenshot
