Hi yogesjoshi,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
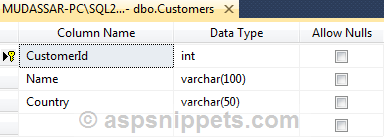
I have already inserted few records in the table.
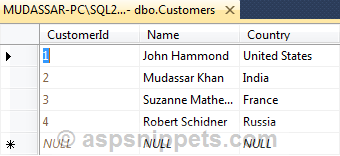
You can download the database table SQL by clicking the download link below.
Download SQL file
Model
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Controller
public class HomeController : Controller
{
private DBCtx Context { get; }
public HomeController(DBCtx _context)
{
this.Context = _context;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public IActionResult GetCustomers()
{
return Json(this.Context.Customers.ToList());
}
[HttpPost]
public IActionResult InsertCustomer([FromBody] Customer customer)
{
this.Context.Customers.Add(customer);
this.Context.SaveChanges();
customer.CustomerId= customer.CustomerId;
return Json(customer);
}
[HttpPost]
public IActionResult DeleteCustomer([FromBody] Customer customer)
{
this.Context.Customers.Remove(customer);
this.Context.SaveChanges();
return Json(customer);
}
}
View
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div style="width: 500px">
<table class="table table-bordered table-striped table-responsive">
<thead>
<tr>
<td>Name</td>
<td><input type="text" id="txtName" class="form-control" /></td>
</tr>
<tr>
<td>Country</td>
<td><input type="text" id="txtCountry" class="form-control" /></td>
</tr>
<tr>
<td colspan="2" align="center">
<input type="button" id="btnAdd" value="Add" class="btn btn-primary" />
</td>
</tr>
</thead>
</table>
<br />
<table id="tblCustomers" cellpadding="0" cellspacing="0" border="1" style="border-collapse:collapse">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Country</th>
<th>Action</th>
</tr>
</thead>
<tbody></tbody>
</table>
</div>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.20/js/jquery.dataTables.min.js"></script>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.20/css/jquery.dataTables.css" />
<script type="text/javascript">
$(function () {
$.ajax({
type: "POST",
url: "/Home/GetCustomers",
data: '{}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
$("#tblCustomers").DataTable({
bLengthChange: true,
lengthMenu: [[5, 10, -1], [5, 10, "All"]],
bFilter: true,
bSort: true,
bPaginate: true,
data: response,
columns: [
{ 'data': 'CustomerId' },
{ 'data': 'Name' },
{ 'data': 'Country' },
{
data: null,
render: function (data, type, row) {
return "<input type='button' id='btnDelete' value='Delete' class='btn btn-danger' data-id='" + data.CustomerId + "' />";
}
}
]
});
},
failure: function (r) {
alert(r.responseText);
},
error: function (r) {
alert(r.responseText);
}
});
$('[id*=btnAdd]').on('click', function () {
var customer = {};
customer.Name = $('[id*=txtName]').val();
customer.Country = $('[id*=txtCountry]').val();
$.ajax({
type: "POST",
url: "/Home/InsertCustomer",
data: JSON.stringify(customer),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
window.location.reload();
}
});
});
$('#tblCustomers tbody').on('click', '[id*=btnDelete]', function () {
if (confirm('Are you sure delete this record?')) {
var customer = {};
customer.CustomerId = $(this).attr('data-id');
$.ajax({
type: "POST",
url: "/Home/DeleteCustomer",
data: JSON.stringify(customer),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
window.location.reload();
}
});
}
});
});
</script>
</body>
</html>
Screenshot
