Hi Jilsoft,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
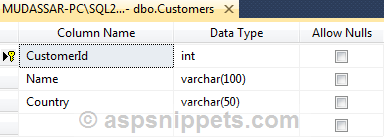
You can download the database table SQL by clicking the download link below.
Download SQL file
HTML
<asp:GridView ID="GridView1" runat="server" ShowFooter="true" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="RowNumber" HeaderText="Row Number" />
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Country">
<ItemTemplate>
<asp:TextBox ID="txtCountry" runat="server"></asp:TextBox>
</ItemTemplate>
<FooterTemplate>
<asp:Button ID="btnAdd" runat="server" Text="Add New Row" OnClick="OnAdd" />
</FooterTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
<asp:Button Text="Save" runat="server" OnClick="OnSave" />
Namespace
C#
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.Data
Imports System.Configuration
Imports System.Data.SqlClient
Code
C#
protected void Page_Load(object sender, EventArgs e)
{
if (!this.IsPostBack)
{
SetInitialRow();
}
}
protected void OnAdd(object sender, EventArgs e)
{
AddNewRowToGrid();
}
protected void OnSave(object sender, EventArgs e)
{
foreach (GridViewRow gvRow in GridView1.Rows)
{
string name = (gvRow.FindControl("txtName") as TextBox).Text;
string country = (gvRow.FindControl("txtCountry") as TextBox).Text;
string conString = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(conString))
{
using (SqlCommand cmd = new SqlCommand("INSERT INTO Customers VALUES (@Name,@Country)", con))
{
cmd.Parameters.AddWithValue("@Name", name);
cmd.Parameters.AddWithValue("@Country", country);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
}
private void AddNewRowToGrid()
{
int rowIndex = 0;
if (ViewState["CurrentTable"] != null)
{
DataTable dtCurrentTable = (DataTable)ViewState["CurrentTable"];
DataRow drCurrentRow = null;
if (dtCurrentTable.Rows.Count > 0)
{
for (int i = 1; i <= dtCurrentTable.Rows.Count; i++)
{
TextBox box2 = (TextBox)GridView1.Rows[rowIndex].FindControl("txtName");
TextBox box3 = (TextBox)GridView1.Rows[rowIndex].FindControl("txtCountry");
drCurrentRow = dtCurrentTable.NewRow();
drCurrentRow["RowNumber"] = i + 1;
dtCurrentTable.Rows[i - 1]["Name"] = box2.Text;
dtCurrentTable.Rows[i - 1]["Country"] = box3.Text;
rowIndex++;
}
dtCurrentTable.Rows.Add(drCurrentRow);
ViewState["CurrentTable"] = dtCurrentTable;
GridView1.DataSource = dtCurrentTable;
GridView1.DataBind();
}
}
SetPreviousData();
}
private void SetPreviousData()
{
int rowIndex = 0;
if (ViewState["CurrentTable"] != null)
{
DataTable dt = (DataTable)ViewState["CurrentTable"];
if (dt.Rows.Count > 0)
{
for (int i = 0; i < dt.Rows.Count; i++)
{
TextBox box2 = (TextBox)GridView1.Rows[rowIndex].FindControl("txtName");
TextBox box3 = (TextBox)GridView1.Rows[rowIndex].FindControl("txtCountry");
box2.Text = dt.Rows[i]["Name"].ToString();
box3.Text = dt.Rows[i]["Country"].ToString();
rowIndex++;
}
}
}
}
private void SetInitialRow()
{
DataTable dt = new DataTable();
DataRow dr = null;
dt.Columns.Add(new DataColumn("RowNumber", typeof(string)));
dt.Columns.Add(new DataColumn("Name", typeof(string)));
dt.Columns.Add(new DataColumn("Country", typeof(string)));
dr = dt.NewRow();
dr["RowNumber"] = 1;
dr["Name"] = string.Empty;
dr["Country"] = string.Empty;
dt.Rows.Add(dr);
ViewState["CurrentTable"] = dt;
GridView1.DataSource = dt;
GridView1.DataBind();
}
VB.Net
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not Me.IsPostBack Then
SetInitialRow()
End If
End Sub
Protected Sub OnAdd(ByVal sender As Object, ByVal e As EventArgs)
AddNewRowToGrid()
End Sub
Protected Sub OnSave(ByVal sender As Object, ByVal e As EventArgs)
For Each gvRow As GridViewRow In GridView1.Rows
Dim name As String = (TryCast(gvRow.FindControl("txtName"), TextBox)).Text
Dim country As String = (TryCast(gvRow.FindControl("txtCountry"), TextBox)).Text
Dim conString As String = ConfigurationManager.ConnectionStrings("constr").ConnectionString
Using con As SqlConnection = New SqlConnection(conString)
Using cmd As SqlCommand = New SqlCommand("INSERT INTO Customers VALUES (@Name,@Country)", con)
cmd.Parameters.AddWithValue("@Name", name)
cmd.Parameters.AddWithValue("@Country", country)
con.Open()
cmd.ExecuteNonQuery()
con.Close()
End Using
End Using
Next
End Sub
Private Sub AddNewRowToGrid()
Dim rowIndex As Integer = 0
If ViewState("CurrentTable") IsNot Nothing Then
Dim dtCurrentTable As DataTable = CType(ViewState("CurrentTable"), DataTable)
Dim drCurrentRow As DataRow = Nothing
If dtCurrentTable.Rows.Count > 0 Then
For i As Integer = 1 To dtCurrentTable.Rows.Count
Dim box2 As TextBox = CType(GridView1.Rows(rowIndex).FindControl("txtName"), TextBox)
Dim box3 As TextBox = CType(GridView1.Rows(rowIndex).FindControl("txtCountry"), TextBox)
drCurrentRow = dtCurrentTable.NewRow()
drCurrentRow("RowNumber") = i + 1
dtCurrentTable.Rows(i - 1)("Name") = box2.Text
dtCurrentTable.Rows(i - 1)("Country") = box3.Text
rowIndex += 1
Next
dtCurrentTable.Rows.Add(drCurrentRow)
ViewState("CurrentTable") = dtCurrentTable
GridView1.DataSource = dtCurrentTable
GridView1.DataBind()
End If
End If
SetPreviousData()
End Sub
Private Sub SetPreviousData()
Dim rowIndex As Integer = 0
If ViewState("CurrentTable") IsNot Nothing Then
Dim dt As DataTable = CType(ViewState("CurrentTable"), DataTable)
If dt.Rows.Count > 0 Then
For i As Integer = 0 To dt.Rows.Count - 1
Dim box2 As TextBox = CType(GridView1.Rows(rowIndex).FindControl("txtName"), TextBox)
Dim box3 As TextBox = CType(GridView1.Rows(rowIndex).FindControl("txtCountry"), TextBox)
box2.Text = dt.Rows(i)("Name").ToString()
box3.Text = dt.Rows(i)("Country").ToString()
rowIndex += 1
Next
End If
End If
End Sub
Private Sub SetInitialRow()
Dim dt As DataTable = New DataTable()
Dim dr As DataRow = Nothing
dt.Columns.Add(New DataColumn("RowNumber", GetType(String)))
dt.Columns.Add(New DataColumn("Name", GetType(String)))
dt.Columns.Add(New DataColumn("Country", GetType(String)))
dr = dt.NewRow()
dr("RowNumber") = 1
dr("Name") = String.Empty
dr("Country") = String.Empty
dt.Rows.Add(dr)
ViewState("CurrentTable") = dt
GridView1.DataSource = dt
GridView1.DataBind()
End Sub
Screenshot
