Hi smile,
Check this example. Now please take its reference and correct your code.
Database
I have made use of the following table Customers with the schema as follows.
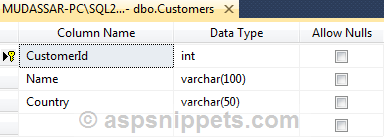
I have already inserted few records in the table.
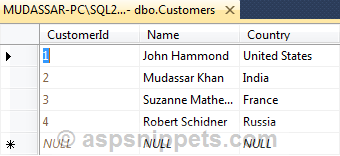
You can download the database table SQL by clicking the download link below.
Download SQL file
Namespaces
C#
using System.Data;
using System.Data.SqlClient;
VB.Net
Imports System.Data
Imports System.Data.SqlClient
Code
C#
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataTable dt;
private void Form1_Load(object sender, EventArgs e)
{
DisplayData();
dt = new DataTable();
dt.Columns.Add("CustomerId");
dt.Columns.Add("Name");
dt.Columns.Add("Country");
dt.Columns.Add("Qty");
}
private void DisplayData()
{
dGVCup.Columns.Clear();
SqlConnection con = new SqlConnection(@"Server=192.168.0.10\SQL2014;DataBase=AjaxSamples;UID=sa;PWD=pass@123");
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "select CustomerId,Name,Country FROM Customers";
cmd.Connection = con;
SqlDataAdapter paging = new SqlDataAdapter();
paging.SelectCommand = cmd;
SqlCommandBuilder sBuilder = new SqlCommandBuilder(paging);
DataSet ds = new DataSet();
paging.Fill(ds, "tblStores");
DataTable sTable = ds.Tables["tblStores"];
dGVCup.DataSource = ds.Tables["tblStores"].DefaultView;
//lblP.Text = dGVCup.Rows.Count.ToString();
if (sTable.Rows.Count < 1)
{
MessageBox.Show("No Record Found", "Information", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
DataGridViewCheckBoxColumn checkBoxColumn = new DataGridViewCheckBoxColumn();
checkBoxColumn.HeaderText = "";
checkBoxColumn.Width = 30;
checkBoxColumn.Name = "checkBoxColumn";
dGVCup.Columns.Insert(0, checkBoxColumn);
dGVCup.SelectionMode = DataGridViewSelectionMode.FullRowSelect;
dGVCup.Columns[1].DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleLeft;
dGVCup.ColumnHeadersDefaultCellStyle.BackColor = System.Drawing.Color.Teal;
dGVCup.ColumnHeadersDefaultCellStyle.ForeColor = System.Drawing.Color.White;
dGVCup.EnableHeadersVisualStyles = false;
//dGVBook.RowHeadersVisible = false;
dGVCup.RowsDefaultCellStyle.BackColor = System.Drawing.Color.White;
dGVCup.AlternatingRowsDefaultCellStyle.BackColor = System.Drawing.Color.White;
dGVCup.CellBorderStyle = DataGridViewCellBorderStyle.Single;
dGVCup.DefaultCellStyle.SelectionBackColor = System.Drawing.Color.LightSeaGreen;
dGVCup.DefaultCellStyle.SelectionForeColor = System.Drawing.Color.White;
dGVCup.DefaultCellStyle.WrapMode = DataGridViewTriState.True;
dGVCup.AllowUserToResizeColumns = true;
}
private void btnCart_Click(object sender, EventArgs e)
{
dGV.Visible = true;
foreach (DataGridViewRow row in dGVCup.Rows)
{
bool isSelected = Convert.ToBoolean(row.Cells["checkBoxColumn"].Value);
if (isSelected)
{
DataRow[] dr = dt.Select("CustomerId='" + row.Cells[1].Value + "'");
if (dr.Length == 0)
{
dt.Rows.Add(row.Cells[1].Value, row.Cells[2].Value, row.Cells[3].Value, "0");
}
}
}
dGV.DataSource = dt;
}
}
VB.Net
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs) Handles MyBase.Load
DisplayData()
dt = New DataTable()
dt.Columns.Add("CustomerId")
dt.Columns.Add("Name")
dt.Columns.Add("Country")
dt.Columns.Add("Qty")
End Sub
Private Sub DisplayData()
dGVCup.Columns.Clear()
Dim con As SqlConnection = New SqlConnection("Server=192.168.0.10\SQL2014;DataBase=AjaxSamples;UID=sa;PWD=pass@123")
Dim cmd As SqlCommand = New SqlCommand()
cmd.CommandText = "select CustomerId,Name,Country FROM Customers"
cmd.Connection = con
Dim paging As SqlDataAdapter = New SqlDataAdapter()
paging.SelectCommand = cmd
Dim sBuilder As SqlCommandBuilder = New SqlCommandBuilder(paging)
Dim ds As DataSet = New DataSet()
paging.Fill(ds, "tblStores")
Dim sTable As DataTable = ds.Tables("tblStores")
dGVCup.DataSource = ds.Tables("tblStores").DefaultView
If sTable.Rows.Count < 1 Then
MessageBox.Show("No Record Found", "Information", MessageBoxButtons.OK, MessageBoxIcon.Information)
Return
End If
Dim checkBoxColumn As DataGridViewCheckBoxColumn = New DataGridViewCheckBoxColumn()
checkBoxColumn.HeaderText = ""
checkBoxColumn.Width = 30
checkBoxColumn.Name = "checkBoxColumn"
dGVCup.Columns.Insert(0, checkBoxColumn)
dGVCup.SelectionMode = DataGridViewSelectionMode.FullRowSelect
dGVCup.Columns(1).DefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleLeft
dGVCup.ColumnHeadersDefaultCellStyle.BackColor = System.Drawing.Color.Teal
dGVCup.ColumnHeadersDefaultCellStyle.ForeColor = System.Drawing.Color.White
dGVCup.EnableHeadersVisualStyles = False
dGVCup.RowsDefaultCellStyle.BackColor = System.Drawing.Color.White
dGVCup.AlternatingRowsDefaultCellStyle.BackColor = System.Drawing.Color.White
dGVCup.CellBorderStyle = DataGridViewCellBorderStyle.Single
dGVCup.DefaultCellStyle.SelectionBackColor = System.Drawing.Color.LightSeaGreen
dGVCup.DefaultCellStyle.SelectionForeColor = System.Drawing.Color.White
dGVCup.DefaultCellStyle.WrapMode = DataGridViewTriState.[True]
dGVCup.AllowUserToResizeColumns = True
End Sub
Private Sub btnCart_Click(ByVal sender As Object, ByVal e As EventArgs) Handles btnCart.Click
dGV.Visible = True
For Each row As DataGridViewRow In dGVCup.Rows
Dim isSelected As Boolean = Convert.ToBoolean(row.Cells("checkBoxColumn").Value)
If isSelected Then
Dim dr As DataRow() = dt.Select("CustomerId='" & row.Cells(1).Value & "'")
If dr.Length = 0 Then
dt.Rows.Add(row.Cells(1).Value, row.Cells(2).Value, row.Cells(3).Value, "0")
End If
End If
Next
dGV.DataSource = dt
End Sub
Screenshot
